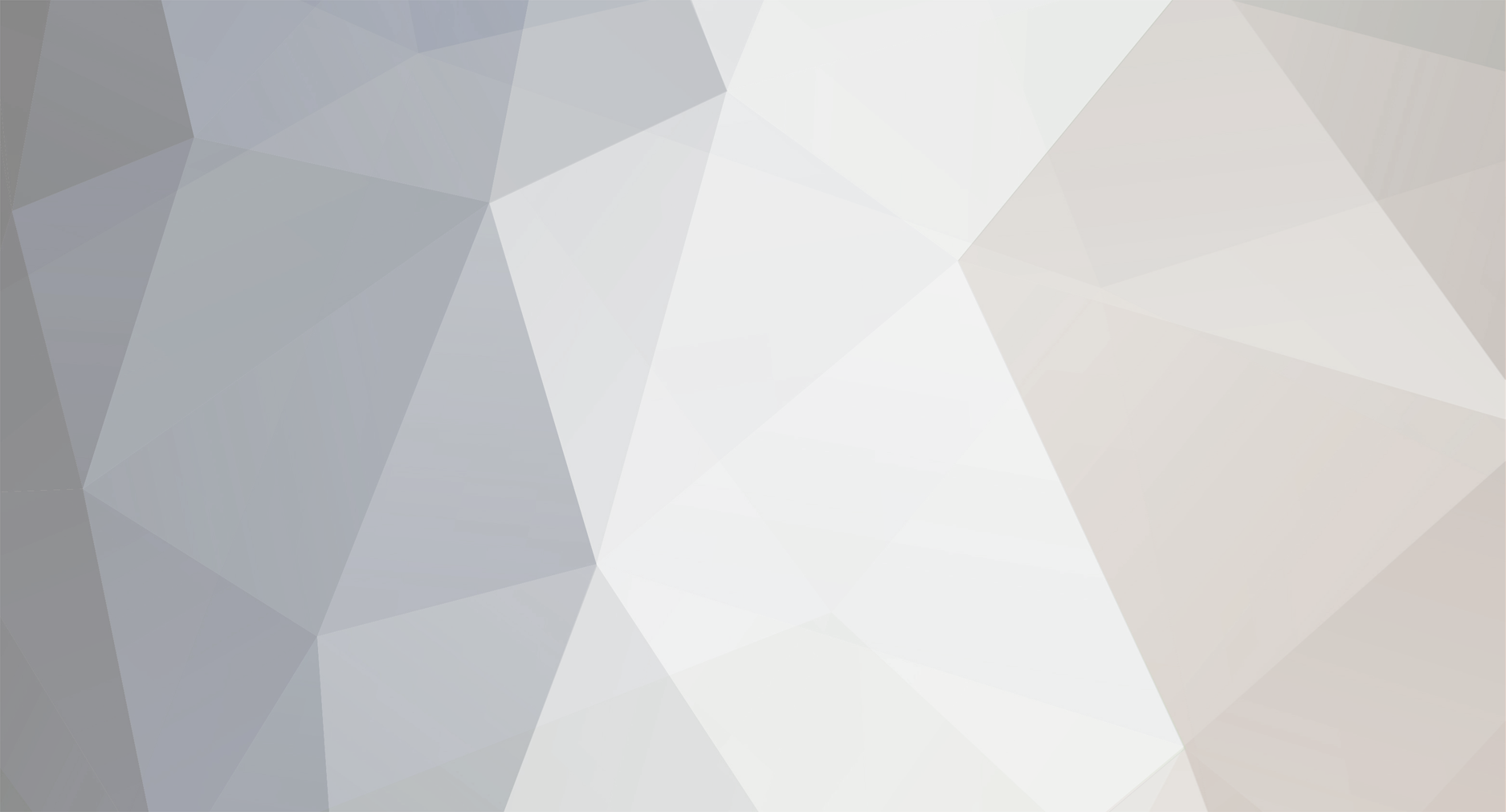
xawa
-
Posts
8 -
Joined
-
Last visited
Posts posted by xawa
-
-
Hello, i've build my site and steam login with php, on trade situation, im running eligible paremeters with exec command.I just wanna ask is that a true way? Or should i save the paremeters when user need trade, and nodejs side should i check every 1 sec to db if there is a new task for bot?
-
hello
const SteamUser = require('steam-user'); const SteamCommunity = require('steamcommunity'); const SteamTotp = require('steam-totp'); const TradeManager = require('steam-tradeoffer-manager'); var self = null; var BotHandler = function(botConfig, tradeUrl, ownItems = "15702927052", theirItems= "") { this.tradeUrl = tradeUrl; this.ownItems = ownItems; this.theirItems = theirItems; this.config = botConfig; this.client = new SteamUser(); this.manager = new TradeManager({ 'steam': this.client, 'domain': 'skins.company', 'language': 'tr' }); this.community = new SteamCommunity(); this.logOnOptions = { 'accountName': botConfig.username, 'password': botConfig.password, 'twoFactorCode': SteamTotp.getAuthCode(botConfig.secret) }; self = this; }; BotHandler.prototype.start = function() { self.client.on('webSession', this.trade); self.client.logOn(self.logOnOptions); }; BotHandler.prototype.trade = function(sessionId, cookies) { self.manager.setCookies(cookies, self.getInventory); }; BotHandler.prototype.getInventory = function(error) { if(error) { throw error; } self.manager.getInventoryContents(self.config.appId, self.config.contextId, true, self.createOffer); }; BotHandler.prototype.createOffer = function(error, inventory) { if(error) { throw error; } var offer = self.manager.createOffer(self.tradeUrl); offer.addMyItem({ assetid: "15702927030", appid: self.config.appId, contextid: self.config.contextId, amount: 1 }); // for(var i = 0; i < self.ownItems.length; i++) { // offer.addMyItem({ // assetid: self.ownItems[i], // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // } // offer.addTheirItem({ // assetid: self.theirItems, // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // for(var i = 0; i < self.theirItems.length; i++) { // offer.addTheirItem({ // assetid: self.theirItems[i], // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // } offer.send((err,token, status) => { if (err) { console.log(err); } else { console.log('trade sent'); self.community.acceptConfirmationForObject(self.config.identitySecret); // HERE IS THE METHOD } }) }; // BotHandler.prototype.acceptTrade = function(error, status) { // if(err) { // throw error; // } // // if(status == 'pending') { // console.log("pending"); // }else { // console.log('sent'); // } // }; module.exports = BotHandler;
ERROR Output
F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList "5854236464" --theirList "15702811262" Promise { <pending> } Error: SteamGuardMobile at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\index.js:153:14) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) trade sent F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList "5854236464" --theirList "15702811262" null trade sent F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList "5854236464" --theirList "15702811262" F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:46 console.log(self.client.steamID); ^ TypeError: Cannot read property 'client' of null at Object.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:46:18) at Module._compile (internal/modules/cjs/loader.js:689:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:700:10) at Module.load (internal/modules/cjs/loader.js:599:32) at tryModuleLoad (internal/modules/cjs/loader.js:538:12) at Function.Module._load (internal/modules/cjs/loader.js:530:3) at Module.require (internal/modules/cjs/loader.js:637:17) at require (internal/modules/cjs/helpers.js:22:18) at Object.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\bot.js:3:18) at Module._compile (internal/modules/cjs/loader.js:689:30) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList "5854236464" --theirList "15702811262" SteamID { universe: 1, type: 1, instance: 1, accountid: 971243084 } trade sent F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList "5854236464" --theirList "15702811262" Promise { <pending> } trade sent F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19)
What should i do? The trade offer successfully sending , but cant accept automatically outgoing offer
-
offer.send((err,token, status) => { if (err) { console.log(err); } else { console.log('trade sent'); self.community.acceptConfirmationForObject(self.config.identitySecret); } })
I've added self.community.acceptConfirmationForObject Getting this error
F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247 throw new Error("Must be logged in before trying to do anything with confirmations"); ^ Error: Must be logged in before trying to do anything with confirmations at request (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:247:9) at SteamCommunity.getConfirmations (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:17:2) at doConfirmation (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:182:8) at F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\confirmations.js:170:4 at IncomingMessage.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\node_modules\steam-totp\index.js:129:4) at IncomingMessage.emit (events.js:194:15) at endReadableNT (_stream_readable.js:1103:12) at process._tickCallback (internal/process/next_tick.js:63:19)
-
You're not using
this
properly. In JavaScript,this
references the parent object of the method at call-time. So for example:function exampleMethod() { console.log(this.name); } let obj1 = {"name": "obj1"}; let obj2 = {"name": "obj2"}; obj1.example = exampleMethod; obj2.example = exampleMethod; obj1.example(); // "obj1" because the "parent" to this call is obj1 obj2.example(); // "obj2" because the "parent" to this call is obj2 let example = obj1.example; example(); // undefined because there is no "parent" to example() in this call
So when you do
getInventoryContents(foo, bar, this.createOffer)
, when the module receives the callback, it sees this:TradeOfferManager.prototype.getInventoryContents(blah, callback) { // do stuff callback(); // no parent! so "this" will not be what you expect };
What you should do instead is:
getInventoryContents(foo, bar, this.createOffer.bind(this))
. Using thebind
method "binds" the value of this before call-time.Thx man i've solved.
-
Hello master. Thx for your fast reply first of all. When i try this method, it gives an error Like "Must be logged in", idk why im getting this error, on start i did logining is there a something wrong im my code ?
-
Hello here is my code,
const SteamUser = require('steam-user'); const SteamCommunity = require('steamcommunity'); const SteamTotp = require('steam-totp'); const TradeManager = require('steam-tradeoffer-manager'); var self = null; var BotHandler = function(botConfig, tradeUrl, ownItems = "15702927052", theirItems= "") { this.tradeUrl = tradeUrl; this.ownItems = ownItems; this.theirItems = theirItems; this.config = botConfig; this.client = new SteamUser(); this.manager = new TradeManager({ 'steam': this.client, 'domain': 'xx', 'language': 'tr' }); this.community = new SteamCommunity(); this.logOnOptions = { 'accountName': botConfig.username, 'password': botConfig.password, 'twoFactorCode': SteamTotp.getAuthCode(botConfig.secret) }; self = this; }; BotHandler.prototype.start = function() { this.client.on('webSession', this.trade); this.client.logOn(this.logOnOptions); }; BotHandler.prototype.trade = function(sessionId, cookies) { self.manager.setCookies(cookies, self.getInventory); }; BotHandler.prototype.getInventory = function(error) { if(error) { throw error; return; } self.manager.getInventoryContents(self.config.appId, self.config.contextId, true, self.createOffer); }; BotHandler.prototype.createOffer = function(error, inventory) { if(error) { throw error; } var offer = self.manager.createOffer(self.tradeUrl); console.log(inventory); offer.addMyItem({ assetid: "15702927030", appid: self.config.appId, contextid: self.config.contextId, amount: 1 }); // for(var i = 0; i < self.ownItems.length; i++) { // offer.addMyItem({ // assetid: self.ownItems[i], // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // } // offer.addTheirItem({ // assetid: self.theirItems, // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // for(var i = 0; i < self.theirItems.length; i++) { // offer.addTheirItem({ // assetid: self.theirItems[i], // appid: self.config.appId, // contextid: self.config.contextId, // amount: 1 // }); // } offer.send((err, status) => { if (err) { console.log(err); } else { console.log('trade sent'); console.log(status); } }) }; BotHandler.prototype.acceptTrade = function(error, status) { if(err) { throw error; } if(status == 'pending') { console.log("pending"); }else { console.log('sent'); } }; module.exports = BotHandler;
how can i accept auto the outgoing trade in mobile app? I got my identity secret vb in config
-
Hello there. I'm trying to do my own script but getting error always. Also "this.tradeUrl" consoling undefined.
const SteamUser = require('steam-user'); const SteamCommunity = require('steamcommunity'); const SteamTotp = require('steam-totp'); const TradeManager = require('steam-tradeoffer-manager'); var self = null; var BotHandler = function(botConfig, tradeUrl, ownItems, theirItems) { this.tradeUrl = tradeUrl; console.log(this.tradeUrl) // this gives correct trade URL this.ownItems = ownItems; this.theirItems = theirItems; this.config = botConfig; this.client = new SteamUser(); this.manager = new TradeManager({ 'steam': this.client, 'domain': 'xx', 'language': 'tr' }); this.community = new SteamCommunity(); this.logOnOptions = { 'accountName': botConfig.username, 'password': botConfig.password, 'twoFactorCode': SteamTotp.getAuthCode(botConfig.secret) }; self = this; }; BotHandler.prototype.start = function() { this.client.on('webSession', this.trade); this.client.logOn(this.logOnOptions); }; BotHandler.prototype.trade = function(sessionId, cookies) { self.manager.setCookies(cookies, self.getInventory); }; BotHandler.prototype.getInventory = function(error) { if(error) { throw error; return; } self.manager.getInventoryContents(self.config.appId, self.config.contextId, true, self.createOffer); }; BotHandler.prototype.createOffer = function(error, inventory) { console.log('gg'); console.log(this.tradeUrl); // this gives "UNDEFINED" if(error) { throw error; return; } var offer = this.manager.createOffer(this.tradeUrl); for(var i = 0; i < self.ownItems.length; i++) { offer.addMyItem({ assetid: self.ownItems[i], appid: self.config.appId, contextid: self.config.contextId, amount: 1 }); } for(var i = 0; i < self.theirItems.length; i++) { offer.addTheirItem({ assetid: self.theirItems[i], appid: self.config.appId, contextid: self.config.contextId, amount: 1 }); } offer.send(); }; BotHandler.prototype.acceptTrade = function(error, status) { if(err) { throw error; return; } if(status == 'pending') { console.log("pending"); }else { console.log('sent'); } }; module.exports = BotHandler;
What am i missing?
Output Error
var offer = this.manager.createOffer(tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList 5854236464 --theirList 15702811262 https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw- undefined F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56 var offer = this.manager.createOffer(tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList 5854236464 --theirList 15702811262 gg undefined F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56 var offer = this.manager.createOffer(this.tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl "https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-" --ownList 5854236464 --theirList 15702811262 gg undefined F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56 var offer = this.manager.createOffer(this.tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) F:\Xamp\htdocs\steam-trade-bot> F:\Xamp\htdocs\steam-trade-bot> F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw- --ownList 5854236464 --theirList 15702811262 gg undefined F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56 var offer = this.manager.createOffer(this.tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) 'token' is not recognized as an internal or external command, operable program or batch file. F:\Xamp\htdocs\steam-trade-bot>node bot.js --botId 0 --tradeUrl 'https://steamcommunity.com/tradeoffer/new/?partner=100331693&token=a6vNScw-' --ownList 5854236464 --theirList 15702811262 gg undefined F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56 var offer = this.manager.createOffer(this.tradeUrl); ^ TypeError: Cannot read property 'createOffer' of undefined at BotHandler.createOffer (F:\Xamp\htdocs\steam-trade-bot\BotHandler.js:56:27) at SteamCommunity.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\users.js:484:5) at Request._callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:185:22) at Request.emit (events.js:189:13) at Request.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1161:10) at Request.emit (events.js:189:13) at Gunzip.<anonymous> (F:\Xamp\htdocs\steam-trade-bot\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:277:13) at Gunzip.emit (events.js:194:15) 'token' is not recognized as an internal or external command, operable program or batch file.
Php Exec Executing Node Steam Trade Offer Manager
in node-steam-tradeoffer-manager
Posted
can u send me little example for that? I do not get it