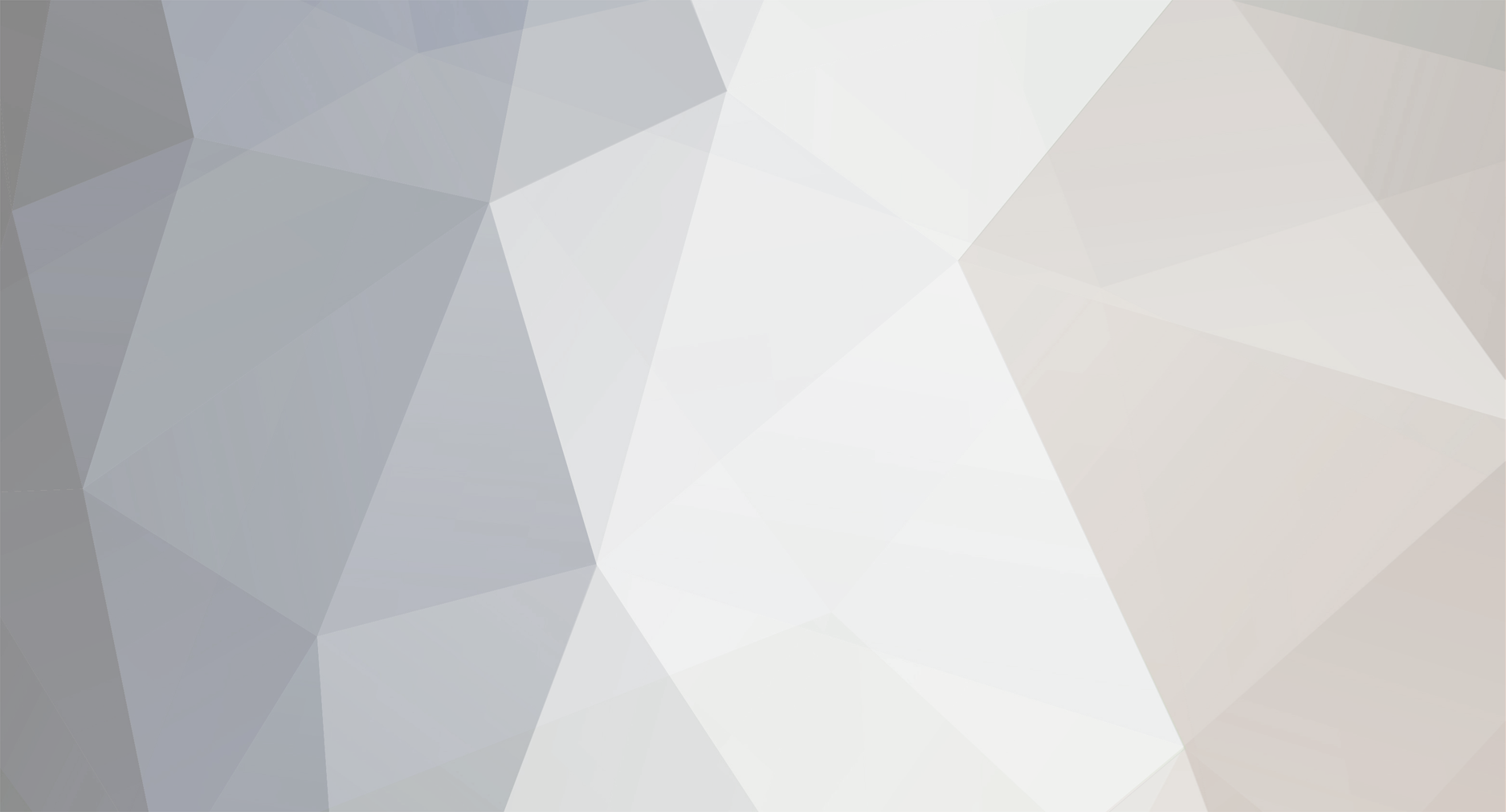
Meshi8
-
Posts
13 -
Joined
-
Last visited
Posts posted by Meshi8
-
-
ConvertToKeys2 is not an async function (nor does it return a Promise), so that's why
await
isn't awaiting anything.What about this?
return new Promise(resolve => {});
Doesn't that mean it returns a promise?
Sry if you facepalm reading this, I'm still trying to wrap my head around async functions
-
Hey,
so Im trying to get the market price of an item I receive on a trade. It works, but for some reason the script won't wait for my async function to finish and returns an empty result
First I have this function which is triggered when I receive an offer for their items
async function ProccessTradeOffer(offer){ let TheirItems = offer.itemsToReceive; const Deposited_Items = await ConvertToKeys2(TheirItems); console.log(Deposited_Items); // this will print null and won't wait for the async to finish }
This is the other function im calling
async function ConvertToKeys2(Items){ let ValueInKeys = []; // array of objects containing info about the items return new Promise(resolve => { setTimeout(() => { if(Market_Value > -1){ obj = { name: item_name, value: Market_Value } ValueInKeys.push(obj); } }, 2000); }); } } return ValueInKeys; }
The problem is that in the main async function it'll not wait for my ConvertToKeys2 function to calculate & add it to array and simply print an empty array.
It's an async function so I don't understand why thisis happening..
Thx for your help
-
Hey,
so I'm trying to get user's personaName in a sent trade offer.
I'm pretty sure I'm doing this right, but I can't figure out what's causing the error..
manager.on("sentOfferChanged", (OFFER, OLDSTATE) => { OFFER.getUserDetails((err, me, them) => { theirname = them.personaName; console.log(theirname); }); });
-
Nickname as in the private name you gave the user, or nickname as in the user's profile (persona) name?
Private name i gave the user
-
Hi,
I'm trying to get a user's nickname.
I know I'm supposed to use getUserDetails and then getPersonas, but what's the nickname property called?
-
Edit: My polllinterval is set to 30 and the problem continues. So I don't think it's Steam that's terminating my connection
-
Hi,
My bot has been working just fine up until yesterday - When it started going offline a few minutes after launch.
I checked the console, and it's not an error on my part, but it says on the console "Killed"
My guess is, the connection is either Killed by Valve (maybe because I send too many requests to their API?) or someone is ddosing/attacking my bot
Here's a screenshot
https://gyazo.com/9a73153c59359b6befddd9ce9d3a63e8
It takes more than a minute for it to shut down.
Important Notes:
I'm hosting my bot on DigitalOcean, and using an SSH connection using putty.
-
-
Hi,
I'd like to get the lowest price of a Background/Emoteicon on the market.
I already figured out how to do that, but for some reason this will ONLY work for anything but Steam items..
Code https://pastebin.com/ujkfYLLa
Also, what's the optimal frequency to use this function? Just so I don't spam it too hard.
-
You have to use getGemValue first because turnItemIntoGems needs the expected value.
That's what I figured.
However, I'm not sure I'm doing this right.
Here's my attempt:
// I'm going through my steam inv with a for loop let MyItem = MySteamInventory[i]; community.getGemValue(MyItem.appid,MyItem.assetid, (res) => { console.log("This Item is worth: "+res.gemValue); });
Am I supposed to use community.getGemValue since getGemValue belongs to node-SteamCommunity?
The Error: This Item is worth: undefined
-
Thanks! I wasn't aware these existed!
However, I'm pretty new to this and really not sure how to use these methods..
Say I have an array called "Selected_Items" with items I filtered myself
I go through it with a for loop and try to convert each item to gems.
I'm supposed to get gemValue first so that I can pass that variable to turnItemIntoGems() method right? I'm not sure how to use getGemValue() method. Could you give me a simple example by any chance?
-
Hi,
is it possible to convert background/emotes into gems with a bot?
Basically, I want the bot to scan it's inventory once a trade is completed, find Backgrounds/Emotes worth 20+ Gems and turn them into Gems.
Is it possible to do?
Getting Market Price (Async Function)
in node-steam-tradeoffer-manager
Posted · Edited by Meshi8
This is what happens:
https://gyazo.com/80c78f9ea22da25b0fa774045e31c27d
It will not wait for the function to finish.
They're both async, it returns a promise but im not sure about the resolve() posittion
Edit: Figuered it out. Just wrapped the entire function with return promise like you suggested, thx for the help