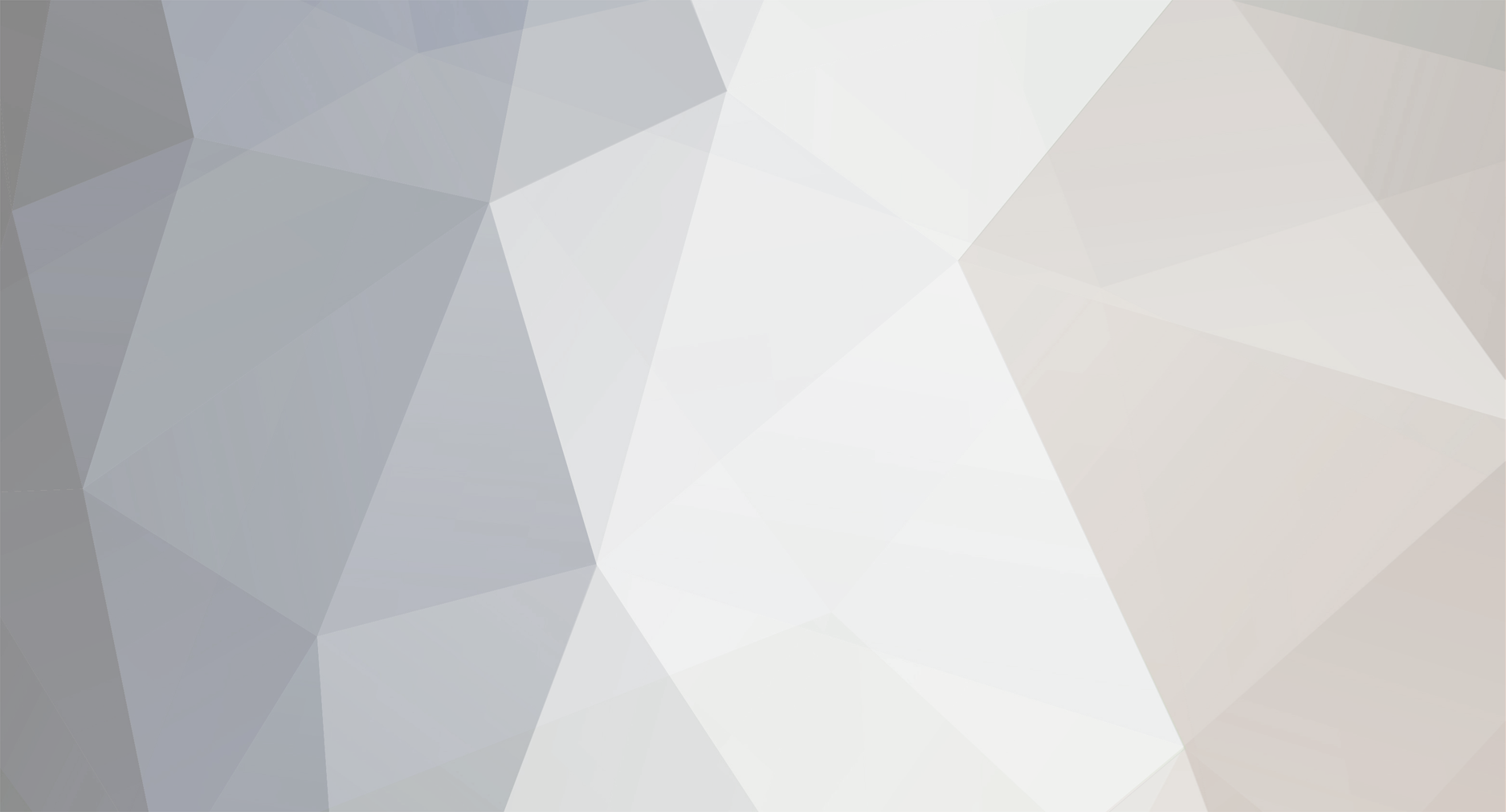
kast0l
-
Posts
12 -
Joined
-
Last visited
Posts posted by kast0l
-
-
Thanks for the answer, one more question, without an api key it’s not possible to use the getOffer method to view information about sent items in a trade?
And if not, is it possible to find out information about a trade without an API key?
-
The trade is successfully sent and confirmed, but then problems arise and I don’t understand why, if the cookies are set correctly
async function SteamTrade(client, account, cookies) {const community = new SteamCommunity();community.setCookies(cookies);const manager = new TradeOfferManager({steam: client,community: community,language: 'en',});await confirmTradeOffer(status, community, account.identity_secret);manager.getOffer(offer.id, (err, offerDetails) => {if (err) {console.error(`${offer.id}: ${err}`);reject(err);} else {console.log(`${offer.id} `);const itemsToGive = offerDetails.itemsToGive;console.log( itemsToGive);resolve({offerDetails, itemsToGive});}});the error occurs after this block of code -
@NickersI figured out what the problem was, I needed to look at the library more carefully, for your case I tested this code and everything was successfully confirmed for me
async function confirmTradeOffers(community, identitySecret) {const time = SteamTotp.time();const confKey = SteamTotp.getConfirmationKey(identitySecret, time, 'conf');const allowKey = SteamTotp.getConfirmationKey(identitySecret, time, 'allow');return new Promise((resolve, reject) => {community.acceptAllConfirmations(time, confKey, allowKey, (err, confs) => {if (err) {console.error(`Ошибка при подтверждении всех трейдов: ${err}`);reject(`Ошибка подтверждения: ${err}`);} else {console.log(`Все трейды успешно подтверждены. Подтверждено трейдов: ${confs.length}`);resolve(`Трейды подтверждены успешно. Подтверждено трейдов: ${confs.length}`);}});});}await confirmTradeOffers(community, account.identity_secret); -
@NickersI found something similar to sda, but which works through node.js, the generation of confirmation codes occurs through steam-totp, I added an account to check if the confirmation works there and the confirmation worked, maybe you can take a look
you'll find something https://github.com/ost056/SteamAuthTool/blob/main/components/master/steam/confirmation.js -
@NickersAny ideas why this works on a server in Europe and doesn’t work on a personal PC in Russia? I’ve just tried everything.And when did this error start appearing?
-
Is there an error in creating confKey?
-
from maFile I took the key after "identity_secret":
-
identitySecret in this format JGhCjFThpoOCuCH4...=,time in this format is 1708401621,data about identitySecret is taken from maFille and they are correct, I checked, since trades are successfully confirmed through SDA,confKey in this format DAgRR7dVxGjC9axkJaf3M...=,offerid also match, but I don't understand why this error occurs Error: Invalid authenticator
here is part of the code that confirms trades and calls this function
async function confirmTradeOffer(community, offer, identitySecret) {const time = SteamTotp.time();const confKey = SteamTotp.getConfirmationKey(identitySecret, time, 'allow');console.log(`Подтверждение трейда с следующими данными:`);console.log(`- ID трейда: ${offer.id}`);console.log(`- Время (Unix Timestamp): ${time}`);console.log(`- Ключ подтверждения: ${confKey}`);console.log(`- IdentitySecret: ${identitySecret}`);return new Promise((resolve, reject) => {community.acceptConfirmationForObject(offer.id, confKey, (err) => {if (err) {console.error(`Ошибка подтверждения трейда ID ${offer.id}: ${err}`);reject(`Ошибка подтверждения трейда: ${err}`);} else {console.log(`Трейд ID ${offer.id} успешно подтвержден.`);resolve('Трейд подтвержден успешно.');}});});}const confirmResult = await confirmTradeOffer(community, offer, account.identitySecret);console.log(confirmResult);break; -
Thanks for the answer
-
After all the code was executed, I noticed that the command line was being entered with a delay, I decided to measure the time and it turned out that Node.js terminates only after a fixed time of 10 seconds has elapsed
-
console output:
Login: starlivovsky5 completed successfullyTotal accounts connected: 1 from 1
All accounts are successfully connected.
Process Node.js ended with code 0
Function execution time: 10.006s
PS C:\Users\Kast0l\Desktop\tetst script>Please help me solve this problem or at least understand what it is
const SteamUser = require("steam-user");const SteamTotp = require("steam-totp");const accounts = require("./accounts.js");let totalConnectedAccounts = 0;const logInAccounts = async () => {for (let account of accounts) {const client = new SteamUserconst logInOptions = {accountName: account.accountName,password: account.password,twoFactorCode: SteamTotp.generateAuthCode(account.sharedSecret)};try {await new Promise((resolve, reject) => {client.logOn(logInOptions);client.once("loggedOn", () => {totalConnectedAccounts++;console.log(`Login: ${account.accountName} completed successfully`);resolve();});client.once("error", (err) => {console.log(`Ошибка при входе для аккаунта ${account.accountName}: ${err}`);reject(err);});});} finally {await client.logOff();}}console.log(`\nTotal accounts connected: ${totalConnectedAccounts} from ${accounts.length}`);console.log("All accounts are successfully connected.");console.time("Function execution time");process.on('exit', (code) => {console.log(`Process Node.js ended with code ${code}`);console.timeEnd("Function execution time");});};logInAccounts()
Question about getOffer and getOffers functions
in node-steam-tradeoffer-manager
Posted
The getOffer and getOffers functions only work if there is a steam web api?