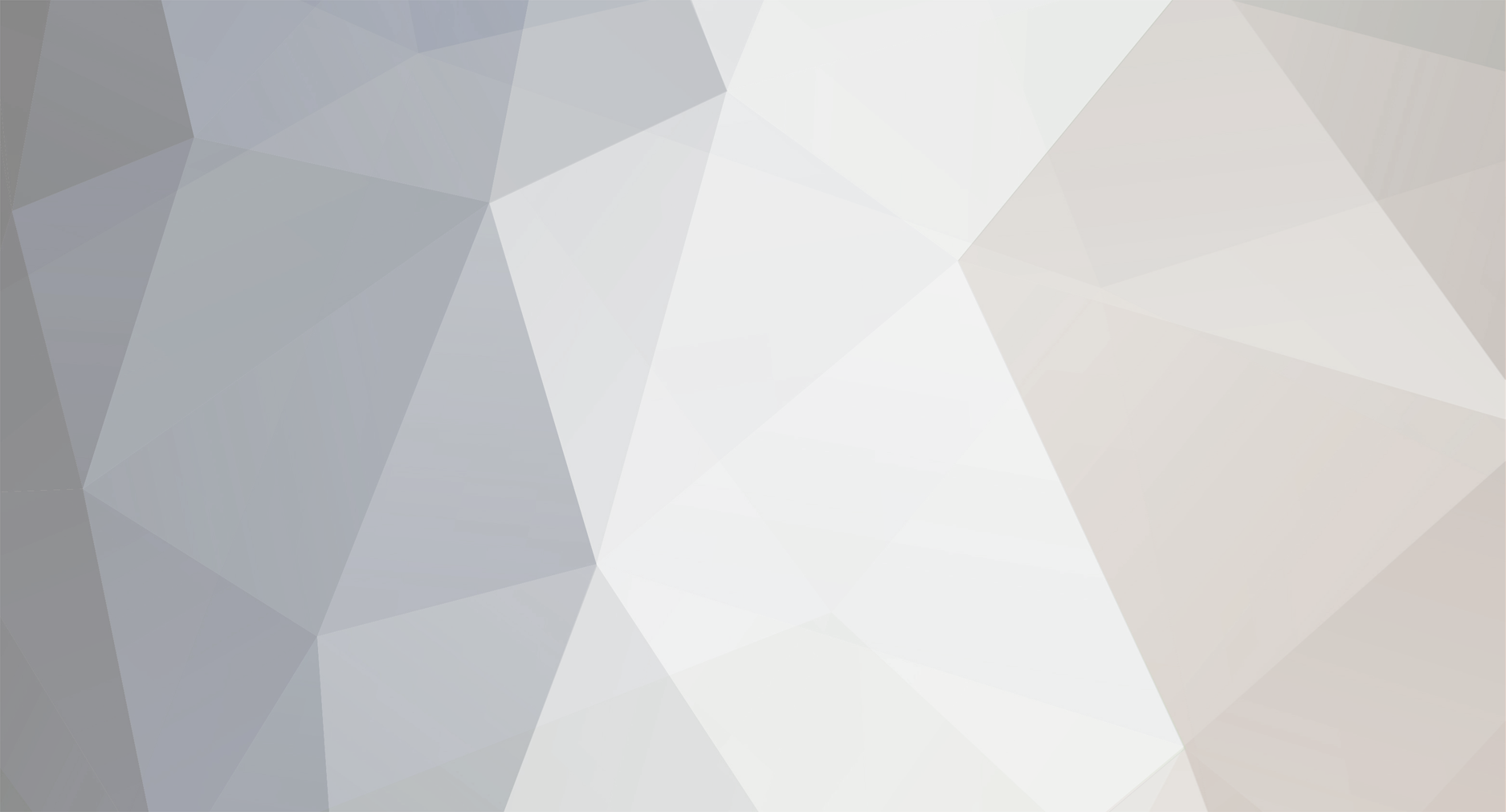
andeh
Member-
Posts
1 -
Joined
-
Last visited
andeh's Achievements
-
Hi! I'm trying to send an offer from one account to another. I'm relative new to programming. Here is the code: var TradeOfferManager = require('steam-tradeoffer-manager'); var SteamID = TradeOfferManager.SteamID; var sid = new SteamID('[MY_STEAM_ID]'); sid.type = SteamID.Type.INDIVIDUAL; sid.instance = SteamID.Instance.DESKTOP; var SteamCommunity = require('steamcommunity'); var SteamTotp = require('steam-totp'); var SteamUser = require('steam-user'); var client = new SteamUser(); var community = new SteamCommunity(); var manager = new TradeOfferManager({ "steam": client, "domain": "MY_DOMAIN", "language": "en" }); // Steam logon options var logOnOptions = { "accountName": "MY_STEAM_USERNAME", "password": "MY_STEAM_PASSWORD", }; client.logOn(logOnOptions); client.on('loggedOn', function() { console.log("Logged into Steam as " + client.steamID.getSteam3RenderedID()); }); // Send offer var offer = manager.createOffer("https://steamcommunity.com/tradeoffer/new/?partner=MY_STEAM_PARTNER&token=MY_STEAM_TOKEN"); manager.loadUserInventory(sid, 730, 2, true, function(err, myItems) { if(err) { console.error(err); return; } console.log(myItems[3]); offer.addMyItem(myItems[3]); offer.setToken("MY_STEAM_TOKEN"); offer.setMessage("Transferring my stuff"); offer.send(function(err) { console.log(err); }); });The output is the following: Logged into Steam as [U:1:MY_STEAM_ID] CEconItem { id: '6763577968', classid: '310778073', instanceid: '302028390', amount: 1, pos: 3, assetid: '6763577968', contextid: '2', appid: '730', icon_url: '-9a81dlWLwJ2UUGcVs_nsVtzdOEdtWwKGZZLQHTxDZ7I56KU0Zwwo4NUX4oFJZEHLbXH5ApeO4YmlhxYQknCRvCo04DEVlxkKgpovrG1eVcwg8zAaAJF_t24nZSOqPrxN7LEmyUB6pwl2r2U84-h2VG1-hA6MWimJ4GRIAU2NAmC-QLvkLjsjJftup3L1zI97T2fu0Hu', icon_url_large: '-9a81dlWLwJ2UUGcVs_nsVtzdOEdtWwKGZZLQHTxDZ7I56KU0Zwwo4NUX4oFJZEHLbXH5ApeO4YmlhxYQknCRvCo04DEVlxkKgpovrG1eVcwg8zAaAJF_t24nZSOqPrxN7LEm1Rd6dd2j6eR99Wh2ADhrRBoYjyiJdXDIAdrYl_UqFK2lL-9hsXt7cvKwHMyvCAg-z-DyIQOpr_N', icon_drag_url: '', name: 'P2000 | Granite Marbleized', market_hash_name: 'P2000 | Granite Marbleized (Field-Tested)', market_name: 'P2000 | Granite Marbleized (Field-Tested)', name_color: 'D2D2D2', background_color: '', type: 'Industrial Grade Pistol', tradable: true, marketable: true, commodity: false, market_tradable_restriction: 7, descriptions: [ { type: 'html', value: 'Exterior: Field-Tested' }, { type: 'html', value: ' ' }, { type: 'html', value: 'Accurate and controllable, the German-made P2000 is a serviceable first-round pistol that works best against unarmored opponents. It has been painted in a marbleized pattern.' }, { type: 'html', value: ' ' }, { type: 'html', value: 'The Italy Collection', color: '9da1a9', app_data: [Object] }, { type: 'html', value: ' ' } ], actions: [ { name: 'Inspect in Game...', link: 'steam://rungame/730/76561202255233023/+csgo_econ_action_preview%20S%owner_steamid%A%assetid%D14285500353088769102' } ], market_actions: [ { name: 'Inspect in Game...', link: 'steam://rungame/730/76561202255233023/+csgo_econ_action_preview%20M%listingid%A%assetid%D14285500353088769102' } ], tags: [ { internal_name: 'CSGO_Type_Pistol', name: 'Pistol', category: 'Type', category_name: 'Type' }, { internal_name: 'weapon_hkp2000', name: 'P2000', category: 'Weapon', category_name: 'Weapon' }, { internal_name: 'set_italy', name: 'The Italy Collection', category: 'ItemSet', category_name: 'Collection' }, { internal_name: 'normal', name: 'Normal', category: 'Quality', category_name: 'Category' }, { internal_name: 'Rarity_Uncommon_Weapon', name: 'Industrial Grade', category: 'Rarity', color: '5e98d9', category_name: 'Quality' }, { internal_name: 'WearCategory2', name: 'Field-Tested', category: 'Exterior', category_name: 'Exterior' } ], is_currency: false, market_marketable_restriction: 0, fraudwarnings: [] } Error: HTTP error 401 at SteamCommunity.manager._community.httpRequestPost (/srv/SteamTrade/node_modules/steam-tradeoffer-manager/lib/classes/TradeOffer.js:477:31) at Request._callback (/srv/SteamTrade/node_modules/steamcommunity/components/http.js:62:14) at Request.self.callback (/srv/SteamTrade/node_modules/request/request.js:187:22) at emitTwo (events.js:106:13) at Request.emit (events.js:191:7) at Request.<anonymous> (/srv/SteamTrade/node_modules/request/request.js:1044:10) at emitOne (events.js:96:13) at Request.emit (events.js:188:7) at IncomingMessage.<anonymous> (/srv/SteamTrade/node_modules/request/request.js:965:12) at emitNone (events.js:91:20)I couldn't get steam-totp to work (getAuthCode() that is), could this be the issue - that the account isn't logged in correctly? Why I suspect this might be the issue is because manager.loadInventory(730, 2, true, function(err, myItems)didn't work, while manager.loadUserInventory(sid, 730, 2, true, function(err, myItems)works. If not, what else could be the issue? Thankful for answers, Andreas
- 1 reply
-
- node.js
- node-steam-tradeoffer-manager
-
(and 1 more)
Tagged with: