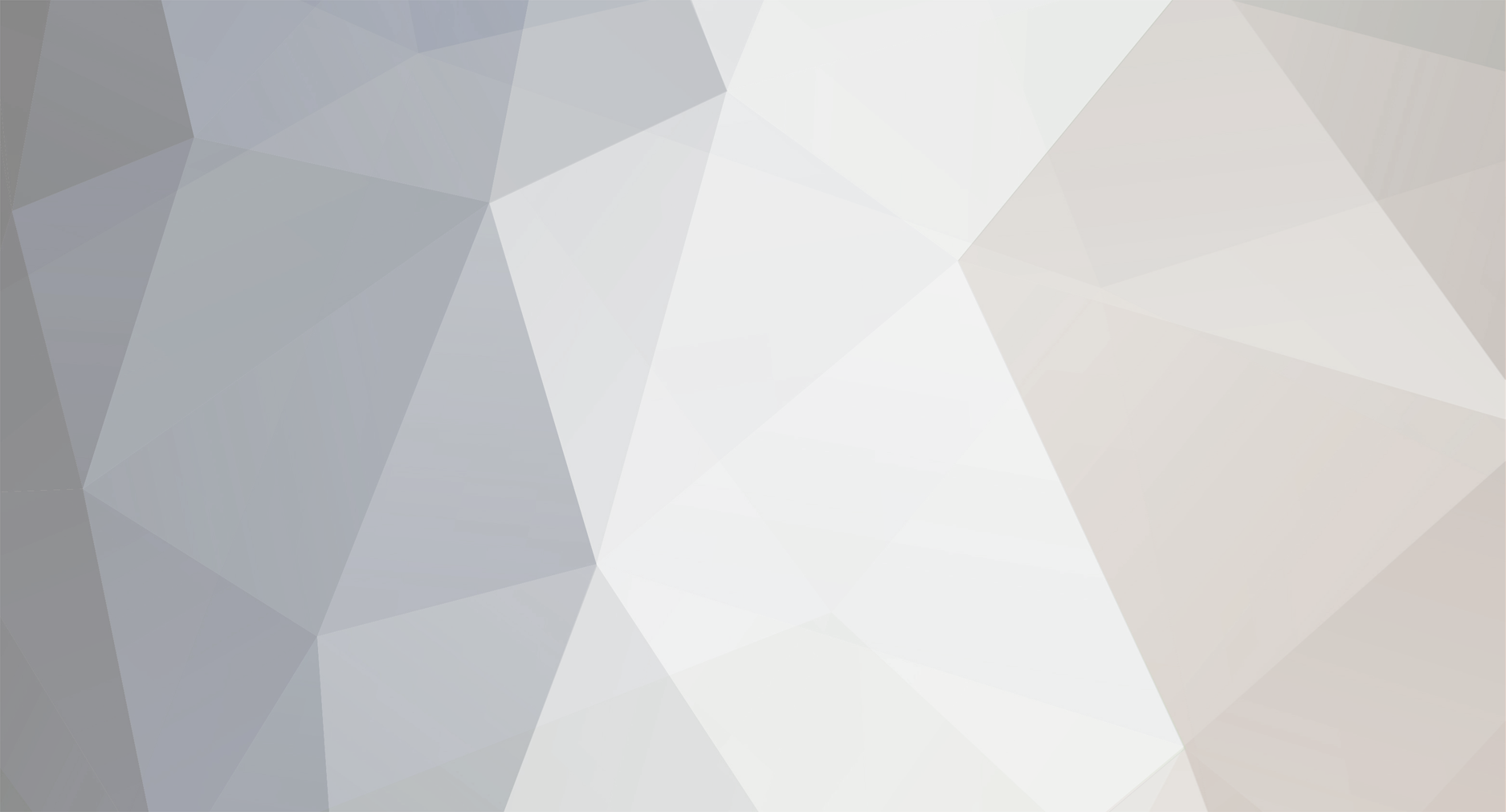
Siezek1
-
Posts
15 -
Joined
-
Last visited
Posts posted by Siezek1
-
-
Hi my bot doesnt accept offers, i dont know why.
My code:
const SteamUser = require('steam-user');
const SteamTotp = require('steam-totp');const SteamCommunity = require('steamcommunity');const TradeOfferManager = require('steam-tradeoffer-manager');const fs = require('fs');const config = require('./config.json');const logOnOptions = {accountName: config.username,password: config.password,twoFactorCode: SteamTotp.generateAuthCode(config.sharedSecret),rememberPassword: true,autoRelogin: true};const client = new SteamUser();const community = new SteamCommunity();const manager = new TradeOfferManager ({steam: client,community: community,language: 'en'});//Logowanie do Steamclient.logOn(logOnOptions);client.on('loggedOn', () => {console.log('Zalogowano!');client.setPersona(SteamUser.EPersonaState.Online);client.gamesPlayed(["🤙Siezek To Kozak! - !komendy🤙",440,570]);});//Komendyclient.on("friendMessage", function(steamID, message) {if (message == "Siema") {client.chatMessage(steamID, "Siema Mordo!");}});client.on("friendMessage", function(steamID, message) {if (message == "!TwĂłrca") {client.chatMessage(steamID, "MĂłj twĂłrca to Siezek --> https://steamcommunity.com/id/siezekYT");}});client.on("friendMessage", function(steamID, message) {if (message == "!twĂłrca") {client.chatMessage(steamID, "MĂłj twĂłrca to Siezek --> https://steamcommunity.com/id/siezekYT");}});client.on("friendMessage", function(steamID, message) {if (message == "!Komendy") {client.chatMessage(steamID, "Moje komendy to:");client.chatMessage(steamID, "1. Siema - Przywitanie siÄ™");client.chatMessage(steamID, "2. !TwĂłrca - Pokazuje mojego twĂłrcÄ™");client.chatMessage(steamID, "3. !yt - Pokazuje kanaĹ‚ moje twĂłrcy");client.chatMessage(steamID, "4. !donate - Donate dla moje twĂłrcy (Wyłączone)");client.chatMessage(steamID, "5. !grupa - Grupa mojego Pana na Steam'ie");client.chatMessage(steamID, "6. !rep - Chcesz +rep? ProszÄ™ bardzo!");}});client.on("friendMessage", function(steamID, message) {if (message == "siema") {client.chatMessage(steamID, "Siema Mordo!");}});client.on("friendMessage", function(steamID, message) {if (message == "!komendy") {client.chatMessage(steamID, "Moje komendy to:");client.chatMessage(steamID, "1. Siema - Przywitanie siÄ™");client.chatMessage(steamID, "2. !TwĂłrca - Pokazuje mojego twĂłrcÄ™");client.chatMessage(steamID, "3. !yt - Pokazuje kanaĹ‚ moje twĂłrcy");client.chatMessage(steamID, "4. !donate - Donate dla moje twĂłrcy (Wyłączone)");client.chatMessage(steamID, "5. !grupa - Grupa mojego Pana na Steam'ie");client.chatMessage(steamID, "6. !rep - Chcesz +rep? ProszÄ™ bardzo!");}});client.on("friendMessage", function(steamID, message) {if (message == "!yt") {client.chatMessage(steamID, "MĂłj kanaĹ‚ na YouTube ->");client.chatMessage(steamID, "https://m.youtube.com/c/SiezekGo");}});client.on("friendMessage", function(steamID, message) {if (message == "!donate") {//client.chatMessage(steamID, "JeĹ›li chcesz wysĹ‚ać donate to masz tutaj tradelink");client.chatMessage(steamID, "Narazie wyłączone");}});client.on("friendMessage", function(steamID, message) {if (message == "!grupa") {client.chatMessage(steamID, "Grupa mojego stwĂłrcy ->");client.chatMessage(steamID, "https://steamcommunity.com/groups/WariatySiezego");}});client.on("friendMessage", function(steamID, message) {if (message == "!rep") {client.chatMessage(steamID, "PoleciaĹ‚ +repik!");community.postUserComment(steamID, "+rep");}});client.on("friendMessage", function(steamID, message) {if (message == "!zmien") {client.chatMessage(steamID, "JuĹĽ zmieniam!");community.changeName('siezek');}});client.on("friendMessage", function(steamID, message) {if (message == "!twicth") {client.chatMessage(steamID, "Twitch mojego twórcy --> http://twitch.tv/siezek");}});//Automatyczne akceptowane do znajomych i grupclient.on('friendRelationship', function(sid, relationship) {if (relationship == SteamUser.EFriendRelationship.RequestRecipient) {console.log("We recieved a friend request from "+sid);client.addFriend(sid, function (err, name) {if (err) {console.log(err);return;}console.log("Accepted user with the name of "+name)})}});client.on('groupRelationship', function(sid, relationship) {if (relationship == SteamUser.EClanRelationship.Invited) {console.log("We were asked to join steam group #"+sid);client.respondToGroupInvite(sid, true);}});client.on('friendsList', function() {for (var sid in client.myFriends);var relationship = client.myFriends[sid]if (relationship == SteamUser.EFriendRelationship.RequestRecipient) {console.log("(offline) We recieved a friend request from "+sid);client.addFriend(sid, function (err, name) {if (err) {console.log(err);return;}console.log("(offline) Accepted user with the name of "+name)})}});client.on('groupList', function() {for (var sid in client.myGroups);var relationship = client.myGroups[sid];if (relationship == SteamUser.EClanRelationship.Invited) {console.log("(offline) We were asked to join steam group #"+sid);client.respondToGroupInvite(sid, true);}})//Akceptowanie i odrzucanie ofertclient.on('webSession', (sid, cookies) => {manager.setCookies(cookies);community.setCookies(cookies);community.startConfirmationChecker(20000, config.identitySecret);});function acceptOffer(offer) {offer.accept((err) => {community.checkConfirmations();console.log("We Accepted an offer");if (err) console.log("There was an error accepting the offer.");});}function declineOffer(offer) {offer.decline((err) => {console.log("We Declined an offer");if (err) console.log("There was an error declining the offer.");});}client.setOption("promptSteamGuardCode", true);manager.on('newOffer', (offer) => {if (offer.partner.getSteamID64() === config.ownerID) {acceptOffer(offer);} else {declineOffer(offer);}}); -
Hi, I will send someone a line to my autobuos, so that the bot will buy me the item as it will be at a given price on steam
-
Ok, thanks
-
Hi, if someone can explain to me / help with translating the bot into another language, I mean that the bot was in two languages, eg the command is !Help and so that it can be translated into another language by command, that is, write the command !En I all commands and bot change language to English and vice versa.
-
Hello, is it possible to write a bot that will receive every day items from Tf2, or bot that will enter the server iddle in Tf2? How can you do a guide to this?
-
For most free games other than TF2, you need to request a license before you can play it.
Thank you man!
-
Hi, I have a bot problem. I can not idolize a few games at once, I can only go to Tf2, if I want another free game and I can not do it, why?
My code:
client.logOn(logOnOptions);
client.on('loggedOn', () => {
console.log('Zalogowano!');
client.setPersona(SteamUser.EPersonaState.Online);
client.gamesPlayed(["Siezek To Kozak!"],440);
});
-
-
-
OK, thank you works, I downloaded any package.json and the bot works. Thank you for your help
-
So I have to change this package.json to package-lock.json and create package.json separately? How would you help me do the package.json?
-
Look, this is my bot. I just deleted the config.json file so that no one would see my login and passwordbotsteam1-master.zip
-
Where should I install it? I already installed it once before putting it on hosting
-
Hi, I did as you asked and did not work out, this is my logssteambot123-console-1563725456224.txtsteambot123-console-1563725562373.txtsteambot123-console-1563728421221.txt
Error when turning on the bot
in General
Posted
I have this problem:
My bot:
bot.js
Config.js
Help pls