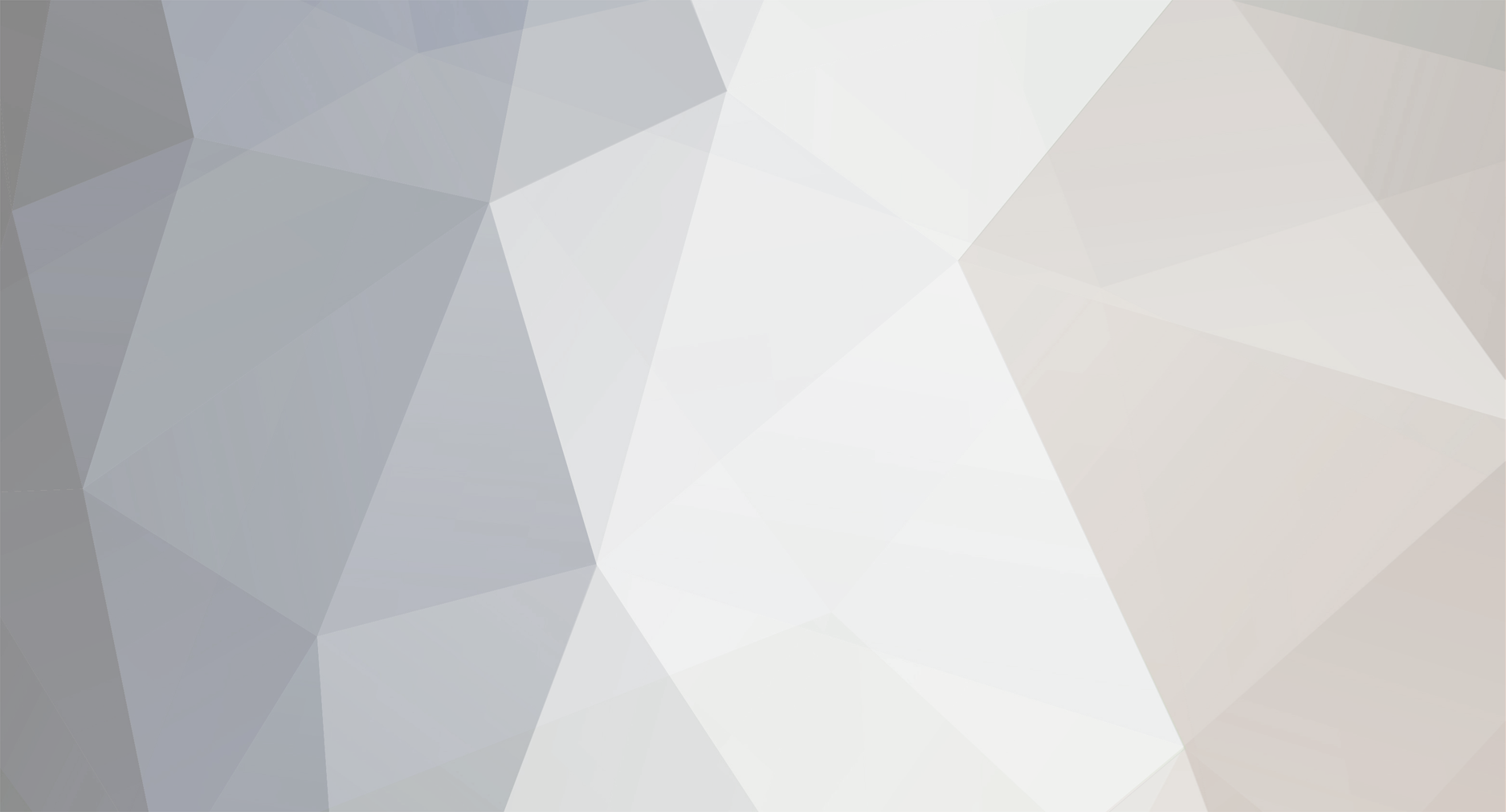
TextDynasty
Member-
Posts
144 -
Joined
-
Last visited
Everything posted by TextDynasty
-
Creating Specific offer from chat command
TextDynasty replied to TextDynasty's topic in node-steam-tradeoffer-manager
The bot now counts each type of metal it requires but it cannot switch to others when one is out of stock. What should I do? -
Creating Specific offer from chat command
TextDynasty replied to TextDynasty's topic in node-steam-tradeoffer-manager
Is it possible to check for users about exchange and convert their metal type if one is running out of stock? -
Creating Specific offer from chat command
TextDynasty replied to TextDynasty's topic in node-steam-tradeoffer-manager
Yes I did something like this but if there is, lets say, not enough scrap metal, the bot cannot proceed the trade because it cannot recognize it can get exchanges from the user. Here is my script so far function peoplechatsell(steamID, name, amount){ Methods.readDataBase('./prices.json') .then((Database) => { if (Database[name] && Database[name].status != 'none' && Database[name] != 'sell') { var item = Database[name]; var stock = item.max_stock; var buy_metal = item.buy.metal * amount; var buy_key = item.buy.keys * amount; var myref = Pures_InStock.metal; var mykey = Pures_InStock.keys; console.log(myref); if(myref >= buy_metal && mykey >= buy_key){ manager.getInventoryContents(440, 2, true, function(err, inventory) { if(err) { console.log('Error getting their inventory: ' + err); }else{ var offer = manager.createOffer(steamID); var key = inventory.filter(function (item) { return item.market_hash_name.match('Mann Co. Supply Crate Key'); }); var ref = inventory.filter(function (item) { return item.market_hash_name.match('Refined Metal'); }); var rec = inventory.filter(function (item) { return item.market_hash_name.match('Reclaimed Metal'); }); var scrap = inventory.filter(function (item) { return item.market_hash_name.match('Scrap Metal'); }); var iteminstock = inventory.filter(function (item) { return item.market_hash_name.match(name); }); var needkey = buy_key; var needref = Math.floor(buy_metal); var a = (buy_metal*10 - needref*10)/3; var needrec = Math.floor(a); var b = buy_metal*10 - needref*10 - needrec*3; var needscrap = Math.floor(b); console.log('Need Key: '+needkey); console.log('Need Ref: '+needref); console.log('Need Rec: '+needrec); console.log('Need Scrap: '+needscrap); console.log(ref.length); console.log(rec.length); console.log(scrap.length); var scrapRequired = Math.floor(buy_metal)*9 +(buy_metal * 10)-(Math.floor(buy_metal)*10); let refRequired = Math.floor(scrapRequired / 9); scrapRequired %= 9; let recRequired = Math.floor(scrapRequired / 3); scrapRequired %= 3; console.log('Need Scrap: '+scrapRequired); console.log('Need Rec: '+recRequired); console.log('Need Ref: '+refRequired); //Check if item is overstock if(amount + iteminstock.length > stock){ console.log('Item overstock'); client.chatMessage(steamID, 'Very sorry, the item ('+name+') you are trying to sell is overstock. The item stock limit is '+stock+'.'); return; }; //Check if there is enough metal to create offer if(key.length >= needkey && ref.length >= needref && rec.length >= needrec && scrap.length >= needscrap){ offer.addMyItems(key.slice(0, needkey)); offer.addMyItems(ref.slice(0, needref)); offer.addMyItems(rec.slice(0, needrec)); offer.addMyItems(scrap.slice(0, needscrap)); } else { console.log('Not enough currencies. Cancelling'); client.chatMessage(steamID, 'Sorry, !sell command only works when bot have enough pure.'); return; } //load user bp manager.getUserInventoryContents(steamID, 440, 2, true, function(err, inventory) { if(err) { console.log('Error getting their inventory: ' + err); client.chatMessage(steamID, 'Sorry, I cannot get your inventory right now.'); }else{ var Item = inventory.filter(function (item) { return item.market_hash_name.match(name); }); if(Item.length >= amount){ offer.addTheirItems(Item.slice(0, amount)); offer.send(function (err, status){ if (err) { console.log(err); } else if (status == 'pending'){ community.acceptConfirmationForObject(config.identity_secret, offer.id, function(err) { if (err) { console.log(err); } else { console.log("Offer confirmed"); client.chatMessage(steamID, 'Trade offer sent.'); } }); } else { console.log('Trade offer #'+offer.id+' sent successfully'); client.chatMessage(steamID, 'Thanks for picking '+config.game+' Service.'); } }); }else{ console.log('User does not have the item.'); client.chatMessage(steamID, 'Please make sure you have the item in your backpack.'); return; } } }) } }) } else { console.log('Not enough currencies to buy.'); client.chatMessage(steamID, 'Sorry, !sell command only works when bot have enough pure.'); return; } } else { console.log('Item not appears on the listings.') client.chatMessage(steamID, 'Sorry, but the item you are selling is not on my buying list.'); return; } }) } -
Creating Specific offer from chat command
TextDynasty replied to TextDynasty's topic in node-steam-tradeoffer-manager
I see. It fixes it now but I want to know if there's any better method to calculate the metal (like check if there's any reclaimed metal and use them if possible instead of all scrap) needed and how to code when there's a need for exchange. My price list is in json format like this: { "Battle-Worn Robot Taunt Processor":{ "sell":{ "keys":0, "metal":0.88 }, "buy":{ "keys":0, "metal":0.77 }, "max_stock":1, "status":"both" } } -
I'd like to create something like !buy and !sell command for my bot but I got error when testing it and I don't know why is that. My code: function peoplechatsell(name){ Methods.readDataBase('./prices.json') .then((Database) => { console.log('hi '+name); if (Database[name]) { var item = Database[name]; var buy_metal = item.buy.metal; var buy_key = item.buy.keys; var myref = Pures_InStock.metal; var mykey = Pures_InStock.keys; console.log(myref) if(myref >= buy_metal && mykey >= buy_key){ console.log('Enough Pure to Buy... Creating Offer'); manager.getInventoryContents(config.bossSteamID, 440, 2, true, function(err, inventory) { if(err) { console.log('Error getting their inventory: ' + err); }else{ var offer = manager.createOffer(config.bossSteamID); var ref = inventory.filter(function (item) { return item.market_hash_name.match('Refined Metal'); }); var scrap = inventory.filter(function (item) { return item.market_hash_name.match('Scrap Metal'); }); var needref = Math.floor(buy_metal); var a = buy_metal*10 - needref*10; var needscrap = Math.floor(a); console.log(needref); console.log(needscrap); offer.addMyItems(ref.slice(0, needref)); offer.addMyItems(scrap.slice(0, needscrap)); manager.getUserInventoryContents(config.bossSteamID, 440, 2, true, function(err, inventory) { if(err) { console.log('Error getting their inventory: ' + err); }else{ var Item = inventory.filter(function (item) { return item.market_hash_name.match(name); }); offer.addTheirItems(Item.slice(0, 1)); offer.send(function (err, status){ if (err) { console.log(err); } else if (status == 'pending'){ community.acceptConfirmationForObject(config.identity_secret, offer.id, function(err) { if (err) { console.log(err); } else { console.log("Offer confirmed"); } }); } else { console.log('Trade offer #'+offer.id+' sent successfully'); } }); } }) } }) } else { console.log('nono'); } } else { return; } }) } const parseAdminMessage = (message) => { if (message == '!update') { updatePrices(); } else if (message == '!code') { var code = SteamTotp.generateAuthCode(config.shared_secret) client.chatMessage(config.bossSteamID, 'Here is the code: ' + code); } else if (message == '!bump') { bumpListings(); } else if (message.indexOf("!sell") === 0) { var name = (message.replace(/^!sell ?/, "")); peoplechatsell(name); } } Error code: hi Battle-Worn Robot Taunt Processor 2 Enough Pure to Buy... Creating Offer C:\Users\User\Desktop\custom-tf2\node_modules\steamcommunity\components\users.js:453 callback(err); ^ TypeError: callback is not a function at C:\Users\User\Desktop\custom-tf2\node_modules\steamcommunity\components\users.js:453:7 at SteamCommunity._checkHttpError (C:\Users\User\Desktop\custom-tf2\node_modules\steamcommunity\components\http.js:110:3) at Request._callback (C:\Users\User\Desktop\custom-tf2\node_modules\steamcommunity\components\http.js:50:61) at Request.self.callback (C:\Users\User\Desktop\custom-tf2\node_modules\request\request.js:185:22) at Request.emit (events.js:198:13) at Request.<anonymous> (C:\Users\User\Desktop\custom-tf2\node_modules\request\request.js:1161:10) at Request.emit (events.js:198:13) at Gunzip.<anonymous> (C:\Users\User\Desktop\custom-tf2\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:286:20) at Gunzip.emit (events.js:203:15) Press any key to continue . . .
-
I've written some lines to check the user background but I don't know how to get all of the items I am receiving and giving. const requestReview = (offer) => { var user = offer.partner.getSteamID64(); for(i = 0; i < offer.itemsToGive.length; i++){ var giveItems = offer.itemsToGive[i].market_hash_name; } for(i = 0; i < offer.itemsToReceive.length; i++){ var receiveItems = offer.itemsToReceive[i].market_hash_name; } client.chatMessage(config.bossSteamID, "--------------------------------"); client.chatMessage(config.bossSteamID, "User "+user+" sent an offer above 14 keys in value. Requesting manual review..."); client.chatMessage(config.bossSteamID, "User Backpack.tf: https://backpack.tf/profiles/"+user); client.chatMessage(config.bossSteamID, "Item to receive: " + receiveItems); client.chatMessage(config.bossSteamID, "Item to give: " + giveItems); client.chatMessage(config.bossSteamID, "Offer ID"); client.chatMessage(config.bossSteamID, offer.id); client.chatMessage(config.bossSteamID, "--------------------------------"); backgroundCheck(offer); } Right now, it would only send me the last item in the trade offer and I would like to modify it to give me all the names in the trade offer. Also is there a way to make it so it can show (ie. 19x keys, 20x ref) instead of all items with just ( , ) separating them (like keys, keys,....)?
-
Thanks that solved it const SteamUser = require('steam-user'); const SteamCommunity = require('steamcommunity'); const SteamTotp = require('steam-totp'); const TradeOfferManager = require('steam-tradeoffer-manager'); const config = require('./config'); let client = new SteamUser(); let manager = new TradeOfferManager({ "steam": client, // Polling every 30 seconds is fine since we get notifications from Steam "domain": "example.com", // Our domain is example.com "language": "en" // We want English item descriptions }); let community = new SteamCommunity(); // Steam logon options let logOnOptions = { "accountName": config.accountName, "password": config.password, "twoFactorCode": SteamTotp.getAuthCode(config.shared_secret) }; client.logOn(logOnOptions); client.on('loggedOn', function() { console.log("Logged into Steam"); }); client.on('webSession', function(sessionID, cookies) { manager.setCookies(cookies, function (err){ if (err) { console.log('Unable to set trade offer cookies: '+err); process.exit(1); // No point in staying up if we can't use trade offers } console.log('Trade offer cookies set.'); }); sendref(); community.setCookies(cookies); }); function sendref() { manager.loadInventory(440, 2, true, function (err, inventory){ if (err) { console.log(err); } else { // I need to send X ref var volume = ; //put whatever amount // Filter out all the ref var ref = inventory.filter(function (item) { return item.market_hash_name.match('Refined Metal') }); // Let the user know we don't have enough ref if (ref.length < volume) { console.log('Not enough ref'); return true; // Give up } // Start a new trade offer var offer = manager.createOffer(config.bossID); // Add what we should to the current trade console.log('Adding '+volume+' metal'); offer.addMyItems(ref.slice(0, volume)) // Send the offer offer.send(function (err, status){ if (err) { console.log(err); } else if (status == 'pending'){ community.acceptConfirmationForObject(config.identity_secret, offer.id, function(err) { if (err) { console.log(err); } else { console.log("Offer confirmed"); } }); } else { console.log('Trade offer sent successfully'); } }); } }); }
-
I modified the code a bit but it always sends me all its refined metal. How can I prevent this and only send a certain amount of metal? function sendref() { manager.loadInventory(440, 2, true, function (err, inventory){ if (err) { console.log(err); } else { // I need to send 5 ref var volume = 5; // Filter out all the ref var ref = inventory.filter(function (item) { return item.market_hash_name.match('Refined Metal') }); // Let the user know we don't have enough ref if (ref.length < volume) { console.log('Not enough ref'); return true; // Give up } // Start a new trade offer var offer = manager.createOffer(config.bossID); // Add what we should to the current trade console.log('Adding '+ref.length+' metal'); offer.addMyItems(ref); // Send the offer offer.send(function (err, status){ if (err) { console.log(err); } else if (status == 'pending'){ community.acceptConfirmationForObject(config.identity_secret, offer.id, function(err) { if (err) { console.log(err); } else { console.log("Offer confirmed"); } }); } else { console.log('Trade offer sent successfully'); } }); } }); }
-
C:\Users\lokin\node_modules\steam-tradeoffer-manager\lib\classes\TradeOffer.js:255 throw new Error("Missing appid, contextid, or assetid parameter"); ^ Error: Missing appid, contextid, or assetid parameter at addItem (C:\Users\lokin\node_modules\steam-tradeoffer-manager\lib\classes\TradeOffer.js:255:9) at TradeOffer.addMyItem (C:\Users\lokin\node_modules\steam-tradeoffer-manager\lib\classes\TradeOffer.js:167:9) at C:\Users\lokin\OneDrive\New folder\bot.js:48:23 at SteamCommunity.<anonymous> (C:\Users\lokin\node_modules\steamcommunity\components\users.js:384:5) at Request._callback (C:\Users\lokin\node_modules\steamcommunity\components\http.js:67:15) at Request.self.callback (C:\Users\lokin\node_modules\request\request.js:185:22) at Request.emit (events.js:182:13) at Request.<anonymous> (C:\Users\lokin\node_modules\request\request.js:1161:10) at Request.emit (events.js:182:13) at Gunzip.<anonymous> (C:\Users\lokin\node_modules\request\request.js:1083:12) Error ^^ I found some examples of creating trade offers on steam but I don't quite understand how to do it. I would like to first differentiate the refined metal from the TF2 inventory and send a certain number (eg 5 refined metal) to the owner when it has more than 5 in its backpack. const SteamUser = require('steam-user'); const SteamCommunity = require('steamcommunity'); const SteamTotp = require('steam-totp'); const TradeOfferManager = require('steam-tradeoffer-manager'); const config = require('./config'); let client = new SteamUser(); let manager = new TradeOfferManager({ "steam": client, // Polling every 30 seconds is fine since we get notifications from Steam "domain": "example.com", // Our domain is example.com "language": "en" // We want English item descriptions }); let community = new SteamCommunity(); // Steam logon options let logOnOptions = { "accountName": config.accountName, "password": config.password, "twoFactorCode": SteamTotp.getAuthCode(config.shared_secret) }; client.logOn(logOnOptions); client.on('loggedOn', function() { console.log("Logged into Steam"); }); client.on('webSession', function(sessionID, cookies) { manager.setCookies(cookies, function() { manager.loadInventory(440, 2, true, function(err, inventory) { if (err) { console.log(err); return; } console.log("Found " + inventory.length + " Team Fortress 2 items"); console.log(inventory); var ref = 0; for (var i = 0; i < inventory.length; i++){ if(inventory[i].market_hash_name == 'Refined Metal'){ ref++ var metal = inventory[i].assetid; } } console.log(metal); if(ref >= 5){ //I want to send 5 metal when the bot backpack has enough metal in its backpack console.log('We have enough refined metal. Sending the trade'); let offer = manager.createOffer(config.bossID); offer.addMyItem(metal); offer.send(function(err, status) { if (err) { console.log(err); return; } if (status == 'pending') { console.log(`Offer #${offer.id} sent, but requires confirmation`); community.acceptConfirmationForObject(config.identity_secret, offer.id, function(err) { if (err) { console.log(err); } else { console.log("Offer confirmed"); } }); } else { console.log(`Offer #${offer.id} sent successfully`); } }); } }); }); community.setCookies(cookies); });
-
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
Is there any example of Promise? -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
I put the return promise to the function I am waiting but cannot resolve the issue const requestReview = (offer) => { var user = offer.partner.getSteamID64(); var score = 0; client.chatMessage(config.bossSteamID, "--------------------------------"); client.chatMessage(config.bossSteamID, "User "+user+" sent an offer above 14 keys in value. Requesting manual review..."); client.chatMessage(config.bossSteamID, "Offer ID"); client.chatMessage(config.bossSteamID, offer.id); client.chatMessage(config.bossSteamID, "--------------------------------"); backgroundCheck(offer,score); } function checkProfile(offer, score) { return new Promise((resolve, reject) => { request('http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key='+config.steamapi+'&steamids='+offer.partner.getSteamID64(), function (error, response, body) { if (response.statusCode == 200){ var obj = JSON.parse(body); var player = obj.response.players; for (var i = 0; i < player.length; i++) { if(player[i].steamid === offer.partner.getSteamID64()){ time = player[i].timecreated; let second = Math.floor(Date.now() / 1000) - player[i].timecreated; let day = second/86400; let month = (day/30).toFixed(); if (isNaN(month) || player == null){ client.chatMessage(config.bossSteamID, 'Cannot find player creation date on Steam.'); }else{ client.chatMessage(config.bossSteamID, 'Created: '+month+' months ago.'); if(month > 6){ score+=1; resolve(score); } } profile = player[i].communityvisibilitystate; if(profile == 1 || profile == 2){ client.chatMessage(config.bossSteamID, 'PRIVATE PROFILE. DO NOT TRADE.'); }else if(profile == 3){ client.chatMessage(config.bossSteamID, 'Profile: Public'); score+=1; resolve(score); } } } }else{ console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }) }); } function checkHours(offer, score) { return new Promise((resolve, reject)=>{ request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key='+config.steamapi+'&include_played_free_games=1&steamid='+offer.partner.getSteamID64(), function (error, response, body) { if(error){ console.log(error); //show errors }else if (response.statusCode == 200){ var obj = JSON.parse(body); if(obj.response.games == null){ console.log('User has private game hour.'); return; }else { var games = obj.response.games; for (var i = 0; i < games.length; i++) { if(games[i].appid == "440"){ var hours = Math.trunc(games[i].playtime_forever/60); var minutes = games[i].playtime_forever % 60; client.chatMessage(config.bossSteamID, 'TF2 time: '+hours+' hours, '+minutes+' minutes.'); if(hours > 500){ score+=1 resolve(score); } } } } }else{ console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }); }) } function checkLevel(offer, score) { return new Promise((resolve, reject)=>{ var user = offer.partner.getSteamID64(); client.getSteamLevels([user], function(requests){ var level = requests[user]; client.chatMessage(config.bossSteamID, 'Steam Level: '+level); if(level > 10){ score+=1; resolve(score); } }); }); } async function backgroundCheck(score){ let score = 0; let score1 = checkHours.resolve(score); let score2 = checkLevel.resolve(score); let score3 = checkProfile.resolve(score); let score = score1+score2+score3; console.log(score); return score; } -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
I still cannot get the scoring system working. I put the commands in sequent though. const requestReview = (offer) => { var user = offer.partner.getSteamID64(); client.chatMessage(config.bossSteamID, "--------------------------------"); client.chatMessage(config.bossSteamID, "User " + user + " sent an offer above 14 keys in value. Requesting manual review..."); client.chatMessage(config.bossSteamID, "Offer ID"); client.chatMessage(config.bossSteamID, offer.id); client.chatMessage(config.bossSteamID, "--------------------------------"); backgroundCheck(offer, score); } function checkProfile(offer, score) { request('http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=' + config.steamapi + '&steamids=' + offer.partner.getSteamID64(), function(error, response, body) { if (error) { console.log(error); //show errors } else if (response.statusCode == 200) { var obj = JSON.parse(body); var player = obj.response.players; for (var i = 0; i < player.length; i++) { if (player[i].steamid === offer.partner.getSteamID64()) { time = player[i].timecreated; let second = Math.floor(Date.now() / 1000) - player[i].timecreated; let day = second / 86400; let month = (day / 30).toFixed(); if (isNaN(month) || player == null) { client.chatMessage(config.bossSteamID, 'Cannot find player creation date on Steam.'); } else { client.chatMessage(config.bossSteamID, 'Created: ' + month + ' months ago.'); if (month > 6) { score += 1; } } profile = player[i].communityvisibilitystate; if (profile == 1 || profile == 2) { client.chatMessage(config.bossSteamID, 'PRIVATE PROFILE. DO NOT TRADE.'); } else if (profile == 3) { client.chatMessage(config.bossSteamID, 'Profile: Public'); score += 1; } } } } else { console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }); } function checkHours(offer, score) { request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key=' + config.steamapi + '&include_played_free_games=1&steamid=' + offer.partner.getSteamID64(), function(error, response, body) { if (error) { console.log(error); //show errors } else if (response.statusCode == 200) { var obj = JSON.parse(body); if (obj.response.games == null) { console.log('User has private game hour.'); return; } else { var games = obj.response.games; for (var i = 0; i < games.length; i++) { if (games[i].appid == "440") { var hours = Math.trunc(games[i].playtime_forever / 60); var minutes = games[i].playtime_forever % 60; client.chatMessage(config.bossSteamID, 'TF2 time: ' + hours + ' hours, ' + minutes + ' minutes.'); if (hours > 500) { score += 1 } } } } } else { console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }); } function checkLevel(offer, score) { var user = offer.partner.getSteamID64(); client.getSteamLevels([user], function(requests) { var level = requests[user]; client.chatMessage(config.bossSteamID, 'Steam Level: ' + level); if (level > 10) { score += 1; } }) } async function backgroundCheck(offer, score) { let score = 0; let score1 = await checkHours(offer, score); let score2 = await checkLevel(offer, score); let score3 = await checkProfile(offer, score); let score = score1 + score2 + score3; console.log(score); return score; } -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
May I know is there a way to make the variable global so each function can change that, instead of making a long function with everything in it? I think it would be better and cleaner this way -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
I added the check status code and it works. So here's my code right now and I am adding a scoring system for background checking, I don't understand why the scores won't add up tho. const requestReview = (offer) => { var user = offer.partner.getSteamID64(); var score = 0 client.chatMessage(config.bossSteamID, "--------------------------------"); client.chatMessage(config.bossSteamID, "User "+user+" sent an offer above 14 keys in value. Requesting manual review..."); client.chatMessage(config.bossSteamID, "Offer ID"); client.chatMessage(config.bossSteamID, offer.id); client.chatMessage(config.bossSteamID, "--------------------------------"); client.getSteamLevels([user], function(requests){ var level = requests[user]; client.chatMessage(config.bossSteamID, 'Steam Level: '+level); if(level > 10){ score+=1; } console.log(score); }); checkProfile(offer, score); checkHours(offer, score); console.log(score); } function checkProfile(offer, score) { request('http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key='+config.steamapi+'&steamids='+offer.partner.getSteamID64(), function (error, response, body) { if(error){ console.log(error); //show errors }else if (response.statusCode == 200){ var obj = JSON.parse(body); var player = obj.response.players; for (var i = 0; i < player.length; i++) { if(player[i].steamid === offer.partner.getSteamID64()){ time = player[i].timecreated; let second = Math.floor(Date.now() / 1000) - player[i].timecreated; let day = second/86400; let month = (day/30).toFixed(); if (isNaN(month) || player == null){ client.chatMessage(config.bossSteamID, 'Cannot find player creation date on Steam.'); }else{ client.chatMessage(config.bossSteamID, 'Created: '+month+' months ago.'); if(month > 6){ score+=1; } } profile = player[i].communityvisibilitystate; if(profile == 1 || profile == 2){ client.chatMessage(config.bossSteamID, 'PRIVATE PROFILE. DO NOT TRADE.'); }else if(profile == 3){ client.chatMessage(config.bossSteamID, 'Profile: Public'); score+=1; } } } }else{ console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }); } function checkHours(offer, score) { request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key='+config.steamapi+'&include_played_free_games=1&steamid='+offer.partner.getSteamID64(), function (error, response, body) { if(error){ console.log(error); //show errors }else if (response.statusCode == 200){ var obj = JSON.parse(body); if(obj.response.games == null){ console.log('User has private game hour.'); return; }else { var games = obj.response.games; for (var i = 0; i < games.length; i++) { if(games[i].appid == "440"){ var hours = Math.trunc(games[i].playtime_forever/60); var minutes = games[i].playtime_forever % 60; client.chatMessage(config.bossSteamID, 'TF2 time: '+hours+' hours, '+minutes+' minutes.'); if(hours > 500){ score+=1 } } } } }else{ console.log(response.statusCode); client.chatMessage(config.bossSteamID, 'Steam cannot load his profile. Require manual check'); } }); } -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
const request = require('request'); const SteamUser = require('steam-user'); const SteamTotp = require('steam-totp'); const TradeOfferManager = require('steam-tradeoffer-manager'); const SteamCommunity = require('steamcommunity'); const config = require('./config'); const client = new SteamUser(); const community = new SteamCommunity(); const manager = new TradeOfferManager({ "steam": client, // Polling every 30 seconds is fine since we get notifications from Steam "domain": "example.com", // Our domain is example.com "language": "en" // We want English item descriptions }); var id = '76561098044947296'; var steamapi = ''; const logOnOptions = { accountName: config.accountName, password: config.password, twoFactorCode: SteamTotp.generateAuthCode(config.shared_secret) }; client.logOn(logOnOptions); client.on('loggedOn', () => { client.setPersona(1); console.log('LogOn'); //check id client.getSteamLevels([id], function(requests){ var level = requests[id]; console.log('Steam Level: '+level); }) }); //Check TF2 hours request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key='+steamapi+'&include_played_free_games=1&steamid='+id, function (error, response, body) { if(error){ console.log(error); //show errors }else { var obj = JSON.parse(body); if(isNaN(obj.response.games) || obj.response.games == null){ console.log('User has private game hour.'); return; }else{ var games = obj.response.games; for (var i = 0; i < games.length; i++) { if(games[i].appid == "440"){ var hours = Math.trunc(games[i].playtime_forever/60); var minutes = games[i].playtime_forever % 60; console.log('TF2 time: '+hours+' hours, '+minutes+' minutes.'); } } } } }); //Check Steam Account Created Time (In months) request('http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key='+steamapi+'&steamids='+id, function (error, response, body) { if(error){ console.log(error); //show errors }else{ var obj = JSON.parse(body); var player = obj.response.players; for (var i = 0; i < player.length; i++) { if(player[i].steamid === id){ time = player[i].timecreated let second = Math.floor(Date.now() / 1000) - player[i].timecreated; let day = second/86400; let month = (day/30).toFixed(); if (isNaN(month) || player == null){ console.log('User has private profile.') }else{ console.log('Account created '+month+' months ago.'); } } } } }); It works fine with many profiles except this one (https://steamcommunity.com/id/76561098044947296/). There's an error said undefined:1 <html> ^ SyntaxError: Unexpected token < in JSON at position 0 at JSON.parse (<anonymous>) at Request._callback (C:\Users\lokin\OneDrive\桌面\New folder\checker.js:44:24) at Request.self.callback (C:\Users\lokin\OneDrive\桌面\New folder\node_modules\request\request.js:185:22) at Request.emit (events.js:182:13) at Request.<anonymous> (C:\Users\lokin\OneDrive\桌面\New folder\node_modules\request\request.js:1161:10) at Request.emit (events.js:182:13) at IncomingMessage.<anonymous> (C:\Users\lokin\OneDrive\桌面\New folder\node_modules\request\request.js:1083:12) at Object.onceWrapper (events.js:273:13) at IncomingMessage.emit (events.js:187:15) at endReadableNT (_stream_readable.js:1094:12) -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
Thanks that solved it. However, I faced another problem. My code right now will show errors when checking private backpack or profile, is there a way to prevent these errors and check these as well? -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
Another thing I would like to check is account creation time. I code a few lines and I would like to find out the current time - creation time to get an estimated time the account history. The problem is I don't know which format is it and how to get the number I wanted. Using this code, I am able to find the user creation time. //Check Steam Account Created Time request('http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key='+steamapi+'&steamids='+id, function (error, response, body) { if(error){ console.log(error); //show errors }else { var obj = JSON.parse(body); var player = obj.response.players; for (var i = 0; i < player.length; i++) { if(player[i].steamid === id){ time = player[i].timecreated console.log(time); var d = new Date(0); // The 0 there is the key, which sets the date to the epoch d.setUTCSeconds(time); console.log(d); } } } }); -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
Thanks. I modified the code a little bit but still cannot get it working. Here is my code currently right now var steamapi = ''; var steamid = ''; request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key='+steamapi+'&include_played_free_games=1&steamid='+steamid, function (error, response, body) { if(error){ console.log(error); }else { var obj = JSON.parse(body); var games = obj.response.games; console.log(games); for (var i = 0; i < games.length; i++) { if(games[i].appid === "440"){ console.log("HI"); }else{ console.log('NO'); //Here I got many NO. I assume it is where the problem starts } } } }); Edit: Fixed. This code is working now request('https://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key='+steamapi+'&include_played_free_games=1&steamid='+steamid, function (error, response, body) { if(error){ console.log(error); }else { var obj = JSON.parse(body); var games = obj.response.games; for (var i = 0; i < games.length; i++) { if(games[i].appid == "440"){ var hours = Math.trunc(games[i].playtime_forever/60); var minutes = games[i].playtime_forever % 60; console.log('User played TF2 for '+hours+' hours, '+minutes+' minutes.'); } } } }); -
Need some help about checking game time
TextDynasty replied to TextDynasty's topic in node-steam-user
I found something like this on the site GetOwnedGames (v0001) Arguments //steamid //The SteamID of the account. //include_appinfo //Include game name and logo information in the output. The default is to return appids only. include_played_free_games By default, free games like Team Fortress 2 are excluded (as technically everyone owns them). If include_played_free_games is set, they will be returned if the player has played them at some point. This is the same behavior as the games list on the Steam Community. format //Output format. json (default), xml or vdf. //appids_filter //You can optionally filter the list to a set of appids. Note that these cannot be passed as a URL parameter, instead you must use the JSON format described in Steam_Web_API#Calling_Service_interfaces. The expected input is an array of integers (in JSON: "appids_filter: [ 440, 500, 550 ]" ) So I tried with steam api key on but I cannot see TF2 in the json. I guess it is because of the "include_played_free_games", but I don't know why isn't it working http://api.steampowered.com/IPlayerService/GetOwnedGames/v0001/?key=XXXXXXXXXXXXXXXXXXXXXX&steamid=76561198302774496&include_played_free_games=true -
So I planned to write a script for the bot to have a background check. Is there a way using steam-user to find out specific user game time? (say like TF2). If so, what is the method? Thanks
-
Need some help about checking Steam level
TextDynasty replied to TextDynasty's topic in node-steam-user
-
Need some help about checking Steam level
TextDynasty replied to TextDynasty's topic in node-steam-user
I edited it a bit const SteamUser = require('steam-user'); const SteamTotp = require('steam-totp'); const config = require('./config'); var client = new SteamUser(); const logOnOptions = { accountName: config.accountName, password: config.password, twoFactorCode: SteamTotp.generateAuthCode(config.shared_secret) }; client.logOn(logOnOptions); client.on('loggedOn', () => { var steamid = '76561198302774496'; client.setPersona(1); console.log('LogOn'); client.getSteamLevels([steamid], function(requests){ var level = requests.steamid; console.log(level); //console.log(requests); works fine but I want it to show the level of the steamid only }) }); -
Need some help about checking Steam level
TextDynasty replied to TextDynasty's topic in node-steam-user
Oh yeah I forgot to log in. But after login, there's still nothing in the console. const SteamUser = require('steam-user'); const SteamTotp = require('steam-totp'); const config = require('./config'); var client = new SteamUser(); const logOnOptions = { accountName: config.accountName, password: config.password, twoFactorCode: SteamTotp.generateAuthCode(config.shared_secret) }; client.logOn(logOnOptions); client.getSteamLevels(['76561198302774496'], function(results){ console.log(results); }) -
So I planned to write a script for the bot to have a background check. const SteamUser = require('steam-user'); var client = new SteamUser(); var steamid = '76561198302774496'; client.getSteamLevels([steamid], function(results){ console.log(results); }) However, it didn't show the results to the console.