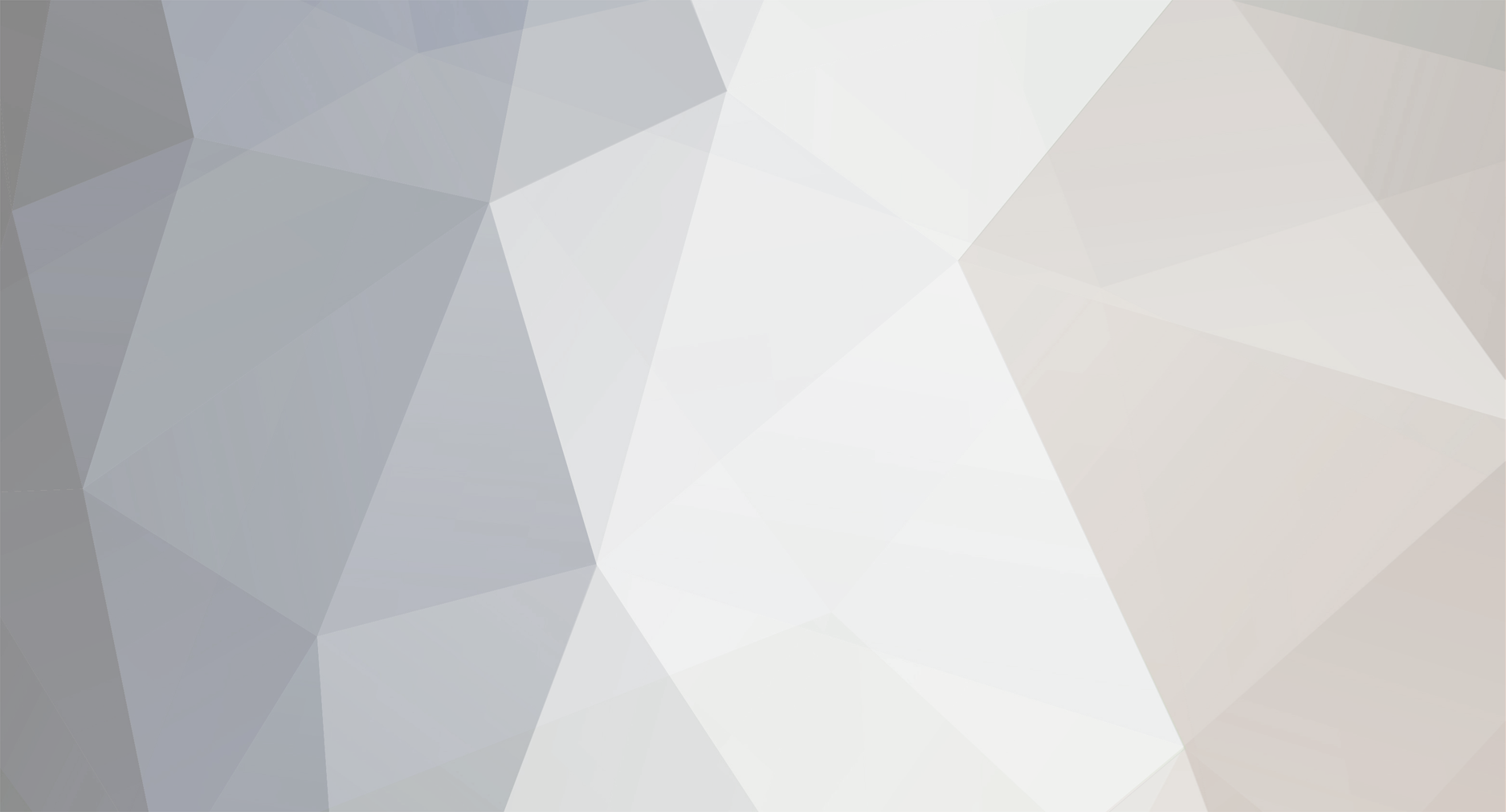
vrtgn
Member-
Posts
61 -
Joined
Everything posted by vrtgn
-
I think it would simply boil down to how you are running the bots, if in the same program, then probably saving to a file, and reading from it (when it needs to be used) would be a good option because keeping inventories in memory, especially large ones, isn't the best of ideas.
-
const url = `https://steamcommunity.com/profiles/${ offer.partner.getSteamID64() }`;
-
Can't get item link from getUserInventoryContents
vrtgn replied to aatmjeets's topic in node-steam-tradeoffer-manager
let link = ""; for (let action of item.actions) { if (action.name === 'Inspect in Game...') { link = action.link; break; } } -
Are you sure offer.partner.getSteam64ID() == config.OwnerId is getting triggered correctly? Or maybe it is and the problem is with your acceptOffer function, I would recommend something like this: function acceptOffer(offer) { offer.accept((err) => { if (err) { console.log('There was an error accepting the offer'); return; } // if we have to give items we have to confirm, otherwise we dont have to if (offer.itemsToGive.length != 0) { community.acceptConfirmationForObject(config.identity_secret, offer.id, (err) => { if (err) { console.log('There was an error confirming the offer'); return; } console.log('Accepted and confirmed the offer'); }) } else { console.log('Accepted the offer'); } }) }
-
No, you should check when you process the offer. It should be among the first things you check in the offer. You can't act on an offer (e.g. decline the offer) after you've accepted it which you are doing in the above code.
-
Yes
-
client.webLogOn(); Sometimes your session can expire, so you can use this event: community.on('sessionExpired', err => client.webLogOn());
-
What do you mean it is timing out? What error does it throw, if any?
-
I think so, you may be able to solve this with polldata.
-
This seems to work, though I'm getting an empty array: client.on('loggedOn', (details) => { console.log("Logged onto Steam as " + client.steamID.getSteam3RenderedID()); client.setPersona(SteamUser.EPersonaState.Online); let steamID = 0; client.getPersonas([steamID]).then(function ({ personas }) { let richPresence = personas[steamID].rich_presence; console.log((richPresence)); }); /* Above is the same as client.getPersonas([steamID]).then(function (obj) { let personas = obj.personas; // code }); */ });
-
// Get all active offers manager.getOffers(TradeOfferManager.EOfferFilter.ActiveOnly, (err, sent, received) => { // Loop through recieved trade offers and execute the processOffer function on each. if (received) { for (var i = 0; i < received.length; i++) { processOffer(received[i]); } } }); You could put the above in a setInterval. The processOffer function is the same thing that you do when you get the newOffer event. So if you have a large function like: manager.on('newOffer', offer => { // code }); You can change it to: manager.on('newOffer', offer => processOffer(offer)); function processOffer(offer) { // code } And so then you can process an offer from elsewhere.
-
acceptConfirmationForObject Sometimes Doesn't Response
vrtgn replied to sergun's topic in node-steam-tradeoffer-manager
What is your code? You may not be error handling. -
how to check if user has atleast 1 active offer
vrtgn replied to DLC-EM_exchangebot's topic in node-steam-tradeoffer-manager
let steamID = "7656"; manager.getOffers(TradeOfferManager.EOfferFilter.Active, (err, sent, recieved) => { sent = sent.filter(offer => offer.partner.getSteamID64() == steamID); // sent.length is equal to how many offers that are currently active have been sent to steamID }) I would use filter on the sent offers received from the getOffers method. -
manager.on('newOffer', offer => { if (offer.itemsToGive.length === 0) { offer.accept(err => err ? console.log('There was an error accpeting the offer!' : null); } });
-
How to use httpRequestPost function to upload an item to the market?
vrtgn replied to eschram's topic in node-steamcommunity
Try adding a header referer to the inventory url.- 2 replies
-
- market listings
- steam api
-
(and 2 more)
Tagged with:
-
Was it because you weren't posting?
-
Try changing the referer to https.
-
You could create a reserve.json file with this sort of structure: { "items": [694868548, 798238548], // asset ids in here, "steamIDToItems": { "7656.......": [694868548], // the users asset ids you have reserved for them, "7656.......": [798238548] } } So when offering items you filter the inventory: inventory.filter(item => !reserve.items.includes(item.assetid)); to get items that are not reserved. And when you need to cancel because someone hasn't accepted the offer in a long time, you get the items you reserved for them from steamIDToItems and remove them as well as removing the IDs from the main items variable: let itemsToRemove = reserve.steamIDToItems[steamID]; reserve.items = reserve.items.filter(item => !itemsToRemove.includes(item)); // remove those items with filter delete reserve.steamIDToItems[steamID]; // delete the reserve items from the file You may want to update the file using fs' writeFile/writeFileSync as these changes are occurring only in memory and will only reflect in the file once you write to the file.
-
Are you passing/setting cookies?
-
I think the first method is better because you only get the inventory from steam when you know it was changed i.e through a trade offer. Second method could get tedious and take long for very large inventories. For your concern of the late sentOfferChanged emit, you could "reserve" those items for the user, so you don't offer them to another user. If the user doesn't accept the offer after a specific amount of time, you tell the user, cancel the offer and unlock those items to offer to somebody else.
-
You want to get the Chat Room Group State of the group, which has a property of members containing an array of Chat Room Member. You could map the SteamID of those members to a single array and check if the sender of the message is included in the mapped array.
-
let groupMembers = [""] // an array containing steamid64's as strings of those group members client.on('friendMessage', (steamID, message) => { if (groupMembers.includes(steamID.getSteamID64())) { // code here } }) Alternatively you could ensure that the only people that can add the bot are the group members and then you wouldn't need the if statement. You may also want to get the group members using some method as if there are new ones you will have to add them manually and for convenience you should store their SteamID64 values in a file, perhaps a .json.