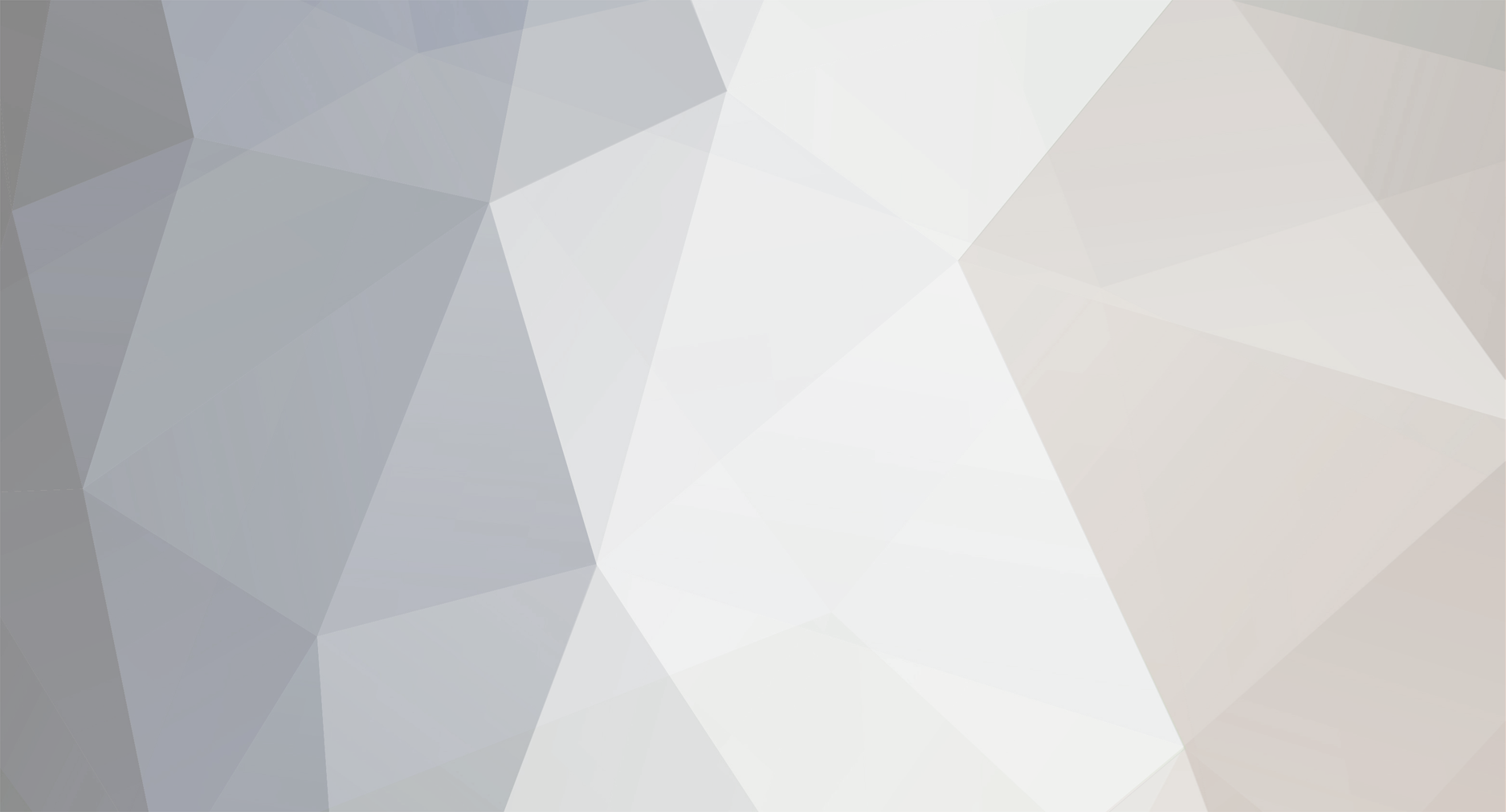
PonyExpress
Member-
Posts
61 -
Joined
-
Last visited
Everything posted by PonyExpress
-
Yes. But I wanted to know what should I do If my next request will use Old endpoint :) (After use new endpoint) In my case I am using the new endpoint until error 429 happens, after that I use the old endpoint.
-
Please advise if I use both methods to get inventory (old and new endpoints). Am I getting startId/startPos correctly? (to continue getting inventory in another way if need) pos = 1 function getItems(inventory, currency, startId, startPos) { // startId - assetid for new endpoint // startPos - pos for old endpoint // ... getting items using the old or new endpoint ... // if used new endpoint: if (body.more_items) { getItems(inventory, currency, body.last_assetid, startPos + body.assets.length) // or startPos should be +/- 1? return } // if used old endpoint: if (body.more) { pos = pos + Object.keys(body.rgInventory).length + Object.keys(body.rgCurrency).length let lastAssetid = Object.keys(body.rgInventory)[Object.keys(body.rgInventory).length - 1] || Object.keys(body.rgCurrency)[Object.keys(body.rgCurrency).length - 1] // this is the last received Assetid? getItems(inventory, currency, lastAssetid, body.more_start) return } }
-
I had this problem. Data from pages like https://steamcommunity.com/profiles/76561198054394425/?xml=1 are cached both in a regular browser and in nodeJS. And as a result, the function getSteamUser can return old data. I don't know how to disable caching in nodeJS. I used quite simply another solution. \node_modules\steamcommunity\classes\CSteamUser.js Tests: community.getSteamUser(new SteamID("76561198125730690"), (error, user) => { if (error) { console.log(error) } else { console.log(user.privacyState) } }) Results, without fix: public // Here I make the profile private public With fix: private // Here I make the profile public public // Here I make the profile for friends only friendsonly
-
"id parameter should be a user URL string or a SteamID object" "id - Either a SteamID object or a user's URL (the part after /id/)" https://github.com/DoctorMcKay/node-steamcommunity/wiki/SteamCommunity#getsteamuserid-callback When trying to use: community.getSteamUser("76561198274802037", (error, user) => { if (error) { console.log(error) } else { console.log(user) } }) I get an error: "The specified profile could not be found." Everything is good when using: new SteamID("76561198274802037") My mistake, sorry, everything is fine. I was sure it was about SteamID64 but here you need a custom url part.
-
Checking card sets on incoming trade offer ?
PonyExpress replied to SENPAY98K's topic in node-steam-tradeoffer-manager
In my case it works like this. Maybe this will help you. // lodash // cards = { "<appid>": ["<classid>": [ {<item>}, ... ], ... ], ... } // cardData = {"220":{"amount":8,"name":"Half-Life 2"} ... } function getSets(cards, callback) { let cardSets = {} let extraCards = [] let unknownCards = [] lodash.forOwn(cards, (cards, id) => { id = id.toString() if (cardData[id]) { let cardCount = lodash.mapValues(cards, array => array.length) cardCount = Object.keys(cardCount).map(number => cardCount[number]) let min = Math.min(...cardCount) let max = Math.max(...cardCount) // There is at least one full set. if (Object.keys(cards).length == cardData[id].amount) { cardSets[id] = [] for (let i = 0; i < min; i++) { let set = [] lodash.forOwn(cards, item => { set.push(item[i]) }) cardSets[id].push(set) } // There are no full sets. } else { min = 0 } // Extra cards: for (let i = min; i < max; i++) { lodash.forOwn(cards, item => { if (item[i]) { extraCards.push(item[i]) } }) } // Unknown cards: } else { lodash.forOwn(cards, item => { unknownCards.push(item) }) } }) callback(cardSets, extraCards, unknownCards) } -
Checking card sets on incoming trade offer ?
PonyExpress replied to SENPAY98K's topic in node-steam-tradeoffer-manager
If I remember correctly, you can specify language in Options ( https://github.com/DoctorMcKay/node-steam-tradeoffer-manager/wiki/TradeOfferManager#language ) In this case, you will receive all data like name and tags. This can take up a lot of memory. (in my case, this required several gigabytes with a large number of trading offers / items in offers) If language disabled, you can check only classid. I don't know of any database that gives any additional information by classid. -
Error: EYldRefreshAppIfNecessary failed with EResult 20 After the trade is completed, I get my Steam inventory using: trade_offer_manager.getUserInventoryContents(my_sid, 753, 6, { tradableOnly: tradableOnly }, (error, inventory) => { //... My inventory is large, ~150k items Earlier (winter-spring), I had a delay of 1 second before getting my inventory and there were no errors. Right now I have a 10 second delay and I still get this error sometimes. If I use less than 8 seconds - the error is always. Is there anything I can do or is this a bug on the steam servers and I can't do anything? Thanks.
-
Is it possible to define a counteroffer?
PonyExpress posted a topic in node-steam-tradeoffer-manager
Hello. 1. If the bot receives a trade offer from user. then the bot makes a counteroffer. 2. If the user makes counteroffer, the bot should decline it. So, The bot must decline offers that are counteroffers. How can I implement this? I did not find any data in TradeOffer object that could help me with this. Are there any ideas how I should do this? Maybe the bot should compare time? Llike if newOffer created time = offer with status "Countered" updated time then decline? Exam;e: let trade = new SteamTradeofferManager() let a trade.on("newOffer", (offer) => { if (offer.created == a) { // decline } else { // create counteroffer } }) trade.on("sentOfferChanged", (offer) => { if (offer.state == 4) { a = offer.updated } }) -
In this code, we just get the last 2 digits of the level, divide it by 10, and round to a smaller one. (example: level 789 = 89 / 10 = 8.9 = 8 = cherry) It does not require special api / packages.
-
// 0 10 20 30 40 50 60 70 80 90 let colors = ["Gray", "Red", "Orange", "Yellow", "Green", "Blue", "Lilac", "Pink", "Cherry", "Brown"]; client.on("friendMessage", (SENDER, MSG) => { client.getSteamLevels([SENDER], (ERR, CURRENTLEVEL) => { if (!ERR) { console.log("The color of your level is " + colors[Math.floor(CURRENTLEVEL[SENDER] % 100 / 10)]); } }); });
-
EYldRefreshAppIfNecessary failed with EResult 55
PonyExpress replied to PonyExpress's topic in node-steam-tradeoffer-manager
Thank you for the information, but I would like to draw attention to this again: 2. In addition, I conducted this experiment several times: let notFriendSID = "76561198042084472"; manager.getUserInventoryContents(notFriendSID, 730, 2, false, (ERR) => { if (ERR) { console.log("getUserInventoryContents ERROR: " + ERR); } else { console.log("getUserInventoryContents OK!"); } }); setTimeout(function () { manager.loadUserInventory(notFriendSID, 730, 2, false, (ERR, INV) => { if (ERR) { console.log("loadUserInventory ERROR: " + ERR); } else { console.log("loadUserInventory OK!"); console.log(INV); } }); }, $SECOND * 3); return; In many cases, I get: Error for getUserInventoryContents and OK for loadUserInventory (after 3 sec). Is this just a coincidence or loadUserInventory is working better? -
In about 50% of the cases when I try to get a CSGO inventory, I get EYldRefreshAppIfNecessary failed with EResult 55. manager.getUserInventoryContents(SID, 730, 2, false, (ERR, USERINVENTORY) => { If I try to open my friends CSGO inventory using Steam, I will also get an error "This inventory is currently unavailable. Please try again later." in about 50% of cases. But, if I offer a trade - the inventory will be available. Why does this happen and can I use another method to get inventory? Left image (profile - inventory), Right image - trade offer. Actually this problem is not permanent. Right now I am not getting this error. But in the screenshot - I first opened the inventory (=error), after that I sent the trade offer (=all good). Reload inventory (=error), Reload trade offer (=all good). I am sure of this and have checked it several times.
-
Will the CS key be tradeable?
PonyExpress replied to PonyExpress's topic in node-steam-tradeoffer-manager
I'll just leave it in case someone needs. // For test: let CONFIG, SENDER = "76561197983852931", amountOfKeys = 10; CONFIG.ACCEPTEDKEYSCS = ["CS20 Case Key", "Prisma Case Key", "Danger Zone Case Key", "Horizon Case Key", "Clutch Case Key", "Spectrum 2 Case Key", "Spectrum Case Key", "Gamma 2 Case Key", "Gamma Case Key", "Glove Case Key", "Chroma 3 Case Key", "Operation Wildfire Case Key", "Falchion Case Key", "Chroma 2 Case Key", "Chroma Case Key", "Operation Vanguard Case Key", "Operation Breakout Case Key", "Huntsman Case Key", "Operation Phoenix Case Key", "Shadow Case Key", "Revolver Case Key", "Winter Offensive Case Key", "eSports Key", "CS:GO Case Key"]; // Getting Inventory: manager.getUserInventoryContents(SENDER, 730, 2, false, (ERR, USERINVENTORY) => { // (!!!) false if (ERR) { console.log(ERR); } else { let hisKeys = [], notMarketable = 0, notTradable = 0; for (let i = 0; i < USERINVENTORY.length; i++) { if (hisKeys.length == amountOfKeys) { break; } else if (CONFIG.ACCEPTEDKEYSCS.includes(USERINVENTORY[i].market_hash_name)) { // This key is accepted if (USERINVENTORY[i].tradable && USERINVENTORY[i].marketable) { hisKeys.push(USERINVENTORY[i]); } else if (!USERINVENTORY[i].marketable) { // This key is not marketable, never notMarketable++; } else if (!USERINVENTORY[i].tradable) { // This key is not tradable, delay up to 7 days notTradable++; } } } // Cancel if the user does not have keys or their quantity is not enough: if (hisKeys.length < amountOfKeys) { let addInfo = ""; if (notTradable) { addInfo += "\n\nSome of your keys (" + notTradable + ") have an exchange delay of up to 7 days."; } if (notMarketable) { addInfo += "\n\nSome of your keys (" + notMarketable + ") bought in the game and can not be exchanged or sold, never!" + "\nYou can return them back within 48 hours after purchase." + "\nhttps://help.steampowered.com/en/wizard/HelpWithGameIssue/?appid=730&issueid=107"; } if (hisKeys.length < 1) { // No one client.chatMessage(SENDER, "You do not have the keys that I accept." + addInfo); } else { // Less than need client.chatMessage(SENDER, "You do not have enough keys." + addInfo); } } else { console.log(hisKeys.length + "/" + amountOfKeys); // trade.addTheirItems(hisKeys); // Adding keys to the trade (!!!) } } }); -
Hey. I wanted to know if there is a way to find out if the User's inventory has been changed? Without a second inventory check. Maybe there is a way to get an inventory hash or item count? Suppose the following situation: User uses command !CHECK The bot receives the user's inventory and gives information. User uses command !SELL The bot receives the user's inventory and and sends the trade offer. I would like to get rid of the second inventory check and use the first (I save it). But there may be another situation: User uses command !CHECK The bot receives the user's inventory and gives information. User receives or sells items / Inventory has been changed User uses command !SELL ? Any ideas? I think I can save the result with a time stamp. But I think that even here rare errors are possible. // !check: community.getUserInventoryContents( ... (err, inv) => { hisInv = [inv, Date.now()]; // !sell: if (hisInv and hisInv[1] < Date.now() - $minute) { //sendtradeoffer } else { community.getUserInventoryContents( ... (err, inv) => { //sendtradeoffer
-
Will the CS key be tradeable?
PonyExpress replied to PonyExpress's topic in node-steam-tradeoffer-manager
Thank you for your quick response. Is there any information about all the properties? I would like to know more about the differences between market_hash_name and market_hash and some others. -
Can I check which key the user has: The key can be tradable in 7 days (Old key) The key was bought in the game and it can not be traded or sold never (New key) Here are the data I get using manager.getUserInventoryContents(FRIEND_SID, 730, 2, false, (ERR, DATA) Old key: CEconItem { appid: 730, contextid: '2', assetid: '18042278743', classid: '3737821870', instanceid: '143865972', amount: 1, pos: 1, id: '18042278743', background_color: '', icon_url: '-9a81dlWLwJ2UUGcVs_nsVtzdOEdtWwKGZZLQHTxDZ7I56KU0Zwwo4NUX4oFJZEHLbXX7gNTPcUxuxpJSXPbQv2S1MDeXkh6LBBOievrLVY2i6ebKDsbv47hw4TTlaSsZeKIxztQu8B03L2Y8Imh2Aftrhc-Z3ezetFDsuzS1g', descriptions: [ { type: 'html', value: 'This key only opens Winter Offensive Cases' }, { type: 'html', value: ' ' }, { type: 'html', value: '', color: '00a000' } ], tradable: false, name: 'Winter Offensive Case Key', name_color: 'D2D2D2', type: 'Base Grade Key', market_name: 'Winter Offensive Case Key', market_hash_name: 'Winter Offensive Case Key', commodity: true, market_tradable_restriction: 7, marketable: true, tags: [ { internal_name: 'CSGO_Tool_WeaponCase_KeyTag', name: 'Key', category: 'Type', color: '', category_name: 'Type' }, { internal_name: 'normal', name: 'Normal', category: 'Quality', color: '', category_name: 'Category' }, { internal_name: 'Rarity_Common', name: 'Base Grade', category: 'Rarity', color: 'b0c3d9', category_name: 'Quality' } ], is_currency: false, market_marketable_restriction: 0, fraudwarnings: [] } UNKNOWN key: CEconItem { appid: 730, contextid: '2', assetid: '17964401838', classid: '3586512622', instanceid: '143865972', amount: 1, pos: 2, id: '17964401838', background_color: '', icon_url: '-9a81dlWLwJ2UUGcVs_nsVtzdOEdtWwKGZZLQHTxDZ7I56KU0Zwwo4NUX4oFJZEHLbXX7gNTPcUxuxpJSXPbQv2S1MDeXkh6LBBOiev8ZQQ30KubIWVDudrgkNncw6-hY-2Fkz1S7JRz2erHodnzig2xqUVvYDrtZNjCAC7WDrU', descriptions: [ { type: 'html', value: 'This key only opens Clutch cases' }, { type: 'html', value: ' ' }, { type: 'html', value: '', color: '00a000' }, { type: 'html', value: ' ' }, { type: 'html', value: '' } ], tradable: false, name: 'Clutch Case Key', name_color: 'D2D2D2', type: 'Base Grade Key', market_name: 'Clutch Case Key', market_hash_name: 'Clutch Case Key', commodity: true, market_tradable_restriction: 7, marketable: false, tags: [ { internal_name: 'CSGO_Tool_WeaponCase_KeyTag', name: 'Key', category: 'Type', color: '', category_name: 'Type' }, { internal_name: 'normal', name: 'Normal', category: 'Quality', color: '', category_name: 'Category' }, { internal_name: 'Rarity_Common', name: 'Base Grade', category: 'Rarity', color: 'b0c3d9', category_name: 'Quality' } ], is_currency: false, market_marketable_restriction: 0, fraudwarnings: [] } As I see, I have to check to see the parameter "marketable"? Should this parameter always be true for all old keys and false for all new keys? Regardless of how the key was received (on the market / trade / purchased in the game long ago). Thanks.
-
Global delay 1sec before client.chatMessage ?
PonyExpress replied to PonyExpress's topic in node-steam-user
Thanks. If anyone wants to solve this problem for their bot, here is a temporary solution: const client = new SteamUser({ "language": "english" }); client.delayMessage = function(sid, msg, type) { setTimeout(function() { client.chatMessage(sid, msg, type); }, 1000); }; // Replace all "client.chatMessage" to "client.delayMessage" -
Hello! In the last 2-3 days there is an (Steam) error when the user does not receive messages from the bot. Messages are successfully sent and will be found in the user's chat after the client reboots. I noticed a pattern: this applies only to messages that are sent instantly (usually such as !help / !prices). The user may not receive such messages. The user will always receive messages with any delay (usually such as !check / !buy) when the bot needs time to make a calculation (However, the user may still not receive messages such as "I'm fulfilling your request, please wait"). I don't want to wait for Steam to fix this. Can I set the minimum delay (at least 1 sec) globally? Test: if (MSG == "QQQ") { client.chatMessage(SENDER, "Ok, QQQ"); } else if (MSG == "WWW") { client.chatTyping(SENDER); client.chatMessage(SENDER, "Ok, WWW"); } else if (MSG == "EEE") { setTimeout(function() { client.chatMessage(SENDER, "Ok, EEE"); }, 1000); } Results, User client: u: QQQ b: <nothing> u: QQQ b: <nothing> u: QQQ b: Ok, QQQ u: QQQ b: <nothing> u: QQQ b: <nothing> u: WWW b: <nothing> u: WWW b: <nothing> u: WWW b: Ok, WWW u: WWW b: <nothing> u: WWW b: <nothing> u: EEE b: Ok, EEE u: EEE b: Ok, EEE u: EEE b: Ok, EEE u: EEE b: Ok, EEE u: EEE b: Ok, EEE PS: I would not want to change ALL client.chatMessage(); to setTimeout(function() { client.chatMessage(); }, 1000); I want to know how can I do this globally in a module file.
-
Thanks. I stopped on this option: function GoTrade(SENDER, MSG, OPTIONS) { // SID, MSG, { OFFER, trade or tradelink } let trade; if (OPTIONS.offerdata) { // trade = OPTIONS.offerdata.counter(); // old trade = OPTIONS.offerdata; // NEW } else if (OPTIONS.tradelink) { //TODO: } else { trade = manager.createOffer(SENDER); } trade.getUserDetails((ERR, BotDetails, USERDETAILS) => { if (ERR) { // ... } else { if (OPTIONS.offerdata) { // NEW trade = OPTIONS.offerdata.counter(); } //...
-
I still have a problem using getUserDetails Should I use getUserDetails before OFFER.counter ? Or maybe i can use for (let trade), which i create? Could you check and tell me the best option that I should use? Thanks! manager.on("newOffer", (OFFER) => { OFFER.itemsToGive = []; // removeTheirItems(OFFER.itemsToReceive); OFFER.itemsToReceive = []; // removeMyItems(OFFER.itemsToGive); //NOTE: Should I do this? // OFFER.getUserDetails((ERR, BotDetails, USERDETAILS) => { // if (ERR) return; // }); //NOTE: Or: // OFFER.getUserDetails((ERR, BotDetails, USERDETAILS) => { // if (!ERR && USERDETAILS.escrowDays <= 3) { // let trade = OFFER.counter(); // GoTrade(OFFER.partner, "trade", {tradedata: trade}); // } // }); GoTrade(OFFER.partner, null, {offerdata: OFFER}); }); client.on("friendMessage", (SENDER, MSG) => { if (MSG == "trade") { GoTrade(SENDER, MSG, {}); } if (MSG.indexOf("https://steamcommunity.com/tradeoffer/new/?partner=") >= 0) { GoTrade(null, null, {tradelink: MSG}); } }); function GoTrade(SENDER, MSG, OPTIONS) { // SID, MSG, { OFFER, trade or tradelink } let trade; if (OPTIONS.offerdata) { trade = OPTIONS.offerdata.counter(); } else if (OPTIONS.tradelink) { //TODO: } else if (OPTIONS.tradedata) { trade = JSON.stringify(tradedata); } else { trade = manager.createOffer(SENDER); } trade.getUserDetails((ERR, BotDetails, USERDETAILS) => { if (ERR) { //NOTE: Should I do this? // if (ERR && !OPTIONS.offerdata) { if (ERR.toString().indexOf("user_inventory_hidden") >= 0) { //... } else if (ERR.toString().indexOf("user_cant_trade") >= 0) { //... } else if (ERR.toString().indexOf("USER_NOT_FRIEND") >= 0) { //... } else { //... } } else if (USERDETAILS.escrowDays > 3) { //... } else { // manager.getUserInventoryContents( ... ) => { // trade.addMyItems( ... ); // trade.addTheirItems( ... ); // trade.setMessage( ... ); // trade.send((ERR) => { } }); }; Also, I don’t understand how it works counter(). How does Steam know if I can send counter or not? Can i send more than one counteroffer usung duplicate()?
-
Yes. I'm trying to simulate the friendMessage event. I want to use it like this: client.on("friendMessage", (SENDER, MSG, FROM_NEW_OFFER, OFFER) => { // or: client.on("friendMessage", (SENDER, [MSG, FROM_NEW_OFFER, OFFER]) => { if (MSG == "help") { // to do something if (FROM_NEW_OFFER) { OFFER.to_do_something(); } } }); manager.on("newOffer", (OFFER) => { client.emit('friendMessage', OFFER.partner, "help", "FROM_NEW_OFFER", OFFER); }); I know I can do it, but I don’t want to: client.on("friendMessage", (SENDER, MSG) => { if (MSG == "help") { Help(SENDER, MSG); } }); manager.on("newOffer", (OFFER) => { Help(OFFER.partner, "help", "FROM_NEW_OFFER", OFFER); }); function Help(SENDER, MSG, FROM_NEW_OFFER, OFFER) { if (MSG == "help") { // to do something if (FROM_NEW_OFFER) { OFFER.to_do_something(); } } }
-
Thank you, it looks like what I need. But I can’t figure out how to do it. client.emit('friendMessage', new SteamID('76561197983852931'), "help");
-
I would like to manually create an event. How should I do this? For example, I want to create an event in which the program will work just like that if it receives a message. I think I should have 2 options: handling multiple event events or creating an event. I think both options should work something like this: var message = {}; client.on("friendMessage", (SENDER, MSG) => { // If an event or variable is not empty: // (client.on("friendMessage", (SENDER, MSG)) || (message != {}) => { if (message) { SENDER = message.msg; MSG = message.sid; message = {}; } client.chatMessage(SENDER, "OK"); }); message = {sid: { universe: 1, type: 1, instance: 1, accountid: 12345678 }, msg: "test"}; // Something like event creation: // client.on("friendMessage", SENDER: { universe: 1, type: 1, instance: 1, accountid: 23587203 }, MSG: "test");
-
Get your trade offer url: community.getTradeURL((ERR, URL) => { if (ERR) { console.log(ERR); } else { console.log(URL); } }); Get another user's trade offer url - I don’t think it is possible. But you can send a counter offer: The user sends you any trading offer You make a counter offer: manager.on("newOffer", (OFFER) => { let trade = OFFER.counter(); Usage example: << Just send me a trade offer by using this link: https://steamcommunity.com/tradeoffer/new/?for_tradingcard=470480_10&partner=192485611&token=Fj1qrxzG . In a few seconds, you will have a new trade offer from me. >> You will not be able to inform the user about the error if he is not in the list of your friends. For this test to work you must have 10-20 cards, public profile, public inventory etc. Otherwise, you will not receive any response.