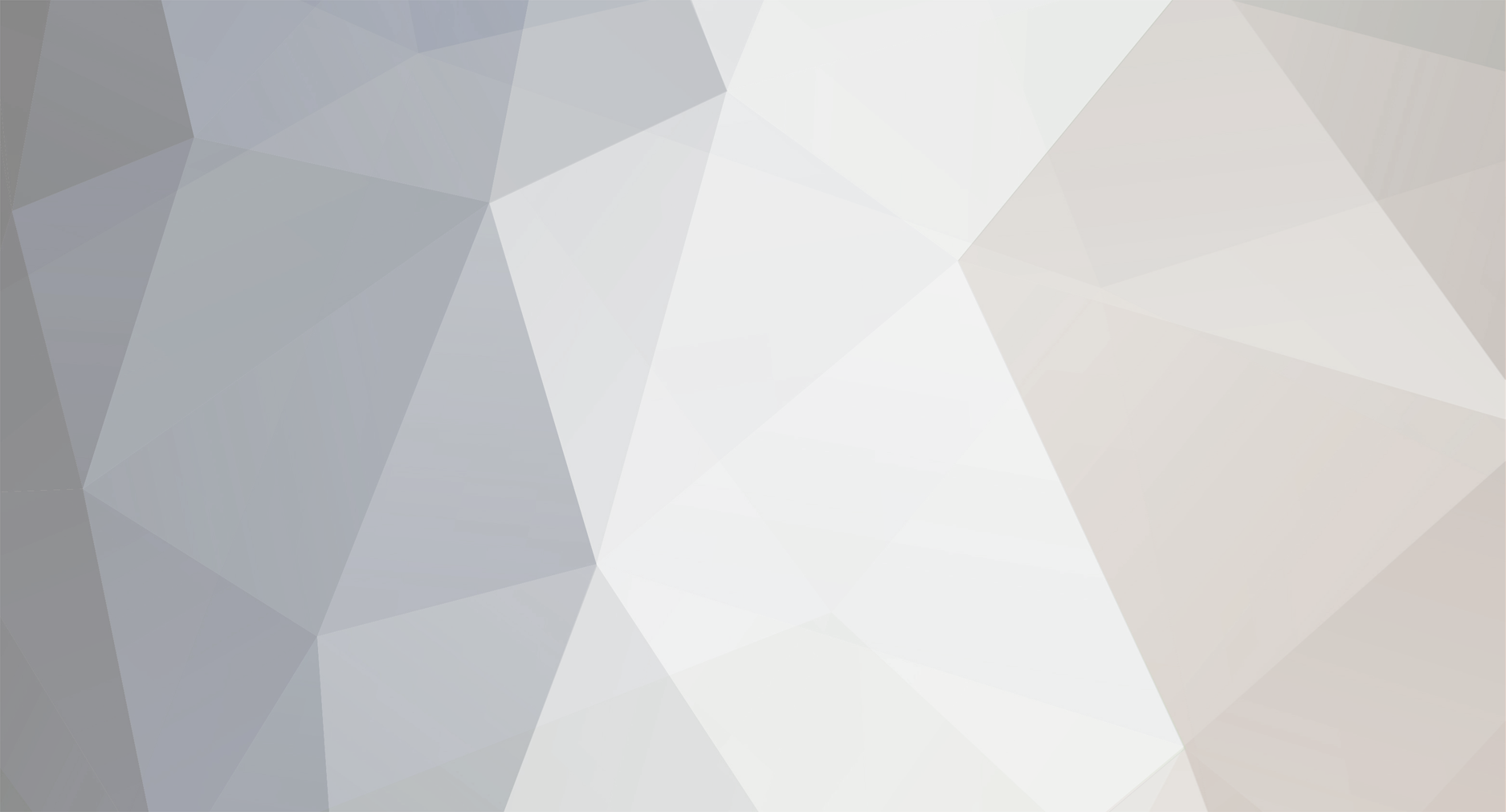
vrtgn
Member-
Posts
61 -
Joined
Everything posted by vrtgn
-
Check if the incoming message is only from those "group members" by using their steam ID 64.
-
You haven't given much detail but I assume your error is Can't resolve 'fs' when bundle with webpack Try putting this in your webpack config: node: { fs: 'empty' }
-
EYldRefreshAppIfNecessary failed with EResult 55
vrtgn replied to PonyExpress's topic in node-steam-tradeoffer-manager
These are both different endpoints, the deprecated loadUserInventory is giving you a response because it is not used as much due to it being unstable. -
You may have made a mistake, getInventoryContents does not have a steam ID param and is used exclusively for fetching the account's inventory, where as getUserInventoryContents like you correctly said is used to fetch other users inventories.
-
I also made this mistake as a beginner. You have a misunderstanding of asynchronous code in javascript. Fetching the inventory takes some time, and so by returning inventory, the variable is being returned before the program can even fetch your inventory. You will have to create your own callback and that works by taking in a function as a callback parameter, and then executing that function once the inventory has been fetched. const getInventory = (callback) => { manager.loadInventory(730, 2, true, (err, inventory) => { if (err) { callback(err); } else { callback(null, inventory); } }) } I personally prefer to check for errors first, but whatever you prefer you can change it to. When we want to fetch the inventory we use it like so: getInventory((err, inventory) => { if (err) { console.log('error getting the inventory :('); } else { // code to do whatever you want with inventory here } }) I also saw that you are using the loadInventory method, it would be much better if you used the getInventoryContents method as it says this in the docs: EDIT: Callbacks are getting ugly nowadays imo so you can also use a promise if you prefer: const getInventory = () => { return new Promise((resolve, reject) => { manager.loadInventory(730, 2, true, (err, inventory) => { if (err) { reject(err); } else { resolve(inventory); } }); }); } getInventory() .then(inventory => { // do whatever here }) .catch(err => console.log('oops')); // OR // this must be inside a async function try { const inventory = await getInventory(); // do whatever here } catch (err) { console.error(err) }
-
How to properly use getUserDetails function
vrtgn replied to IdiNium's topic in node-steam-tradeoffer-manager
@IdiNium Oh damn, I never realised that there are two separate functions for review offer and trade summary function but now I come to think of it it makes sense why. Do you have any idea how I can enable discord webhooks to my account to test this feature and give you a solid answer rather than just guessing? btw ts makes me cry lol @IdiNium Hey, just looked over your code and saw that in the getPartnerDetails function in getSteamUser callback you return the object with the property personName rather than personaName. In addition, in the sendSummary you assign partnerAvatar to details.personaName rather than details.avatarFull. You may also want to if else in the summary as you are assigning the values of partnerName and partnerAvatar but returning before you send them. -
How to properly use getUserDetails function
vrtgn replied to IdiNium's topic in node-steam-tradeoffer-manager
The CSteamUser is a class, but as the docs say: Instantiated in other words means created like you have done: const sComm = new CSteamUser(); The only way to get it is by using the getSteamUser call. Actually you can assign methods to the steamcommunity class using prototype based inheritance. Essentially we can refer to the steamcommunity class from another file and give it a method that we want and if you look at the CSteamUser.js file you can see that steamcommunity is bought in and assigned the getSteamUser method: var SteamCommunity = require('../index.js'); ... SteamCommunity.prototype.getSteamUser = function(id, callback) {...} BUT, you say the file is modified (and yes it was made by Nick) instead it seems like (Source) So the file is a sort of skeleton/description about the steamcommunity module. I'm assuming the methods defined in that file are only methods the bot needs to use. So if that is the case then we can add the getSteamUser method in that file: getSteamUser(id: SteamID | string, callback: (err?: Error, user?: any) => void): void; For simplicity and to be on the safe side Im going to keep user as any but you could make it a type of SteamCommunity.User but you will have to add the SteamCommunity.User interface in the SteamCommunity namespace: interface User { name: string; getAvatarURL: (size: string) => void; } I rarely use TS so this maybe wrong but let me know how it goes. -
How to properly use getUserDetails function
vrtgn replied to IdiNium's topic in node-steam-tradeoffer-manager
You can make the function a private and place it in the body of the MyHandler, but you will have to call it by prefixing "this." before the function. this.getPartnerDetails(...) As for the "this" error you can change the parameters of the getPartnerDetails to: function getPartnerDetails(this: any, offer: TradeOfferManager.TradeOffer, callback: (err: any, details: any) => void): any { -
How to properly use getUserDetails function
vrtgn replied to IdiNium's topic in node-steam-tradeoffer-manager
Happy to help, but note that a function is assignable to a variable; you just can't return that value from the function. You will progress as you learn. I'll be using callbacks below so here is a tutorial/explanation if you still don't entirely understand. I'm not entirely sure how the Discord webhooks work, I myself have never used them. But from what it seems is that if an offer is active you can get the details of the users, however if it is not, then you will have to get it in some other way. Luckily for us, the offer class has a state property for us to check whether the offer is "Active". First, let's start by making the a separate function to get the partner's name and avatar. function getPartnerDetails(offer: TradeOfferManager.TradeOffer, callback: (err: any, details: any): void): any { // check state of the offer if (offer.state == TradeOfferManager.ETradeOfferState.Active) { offer.getUserDetails(function(err, me, them) { if (err) { callback(err, {}); // idk if tsc will complain if theres no second param } else { callback(null, them); } }); } else { this.bot.community.getSteamUser(offer.partner, (err, user) => { if (err) { callback(err, {}); } else { callback(null, { personaName: user.name, avatarFull: user.getAvatarURL('full') }); } }); } } If the offer is active we obtain the details through the get user details method. Otherwise, we utilise getSteamUser from steamcommunity which returns a CSteamUser object which we can extract the personaName and avatarFull from and send it back. Now when we want to use it we simply do: let personaName; let avatarFull; getPartnerDetails(offer, function(err, details) { if (err) { log.debug('Error getting partner details, returning ? avatar and unknown name'); personaName = 'unknown'; avatarFull = 'https://p7.hiclipart.com/preview/313/980/1020/question-mark-icon-question-mark-png.jpg'; } else { log.debug('Successfully obtained partner details'); personaName = details.personaName; avatarFull = details.avatarFull; } const stringified = JSON.stringify(discordTradeSummary) .replace(/%partnerId%/g, partnerSteamID) .replace(/%partnerName%/g, partnerName) .replace(/%partnerAvatar%/g, partnerAvatar) .replace(/%offerId%/g, offer.id) .replace(/%tradeNum%/g, tradesMade.toString()) .replace(/%tradeSummary%/g, tradeSummary.replace('Offered:', '\\n Offered:')) .replace(/%currentTime%/g, moment().format('MMMM Do YYYY, HH:mm:ss') + ' UTC'); const jsonObject = JSON.parse(stringified); request.send(JSON.stringify(jsonObject)); }); and so now the sendWebHookTradeSummary looks like private sendWebHookTradeSummary(offer: TradeOfferManager.TradeOffer): void { const request = new XMLHttpRequest(); request.open('POST', process.env.DISCORD_WEBHOOK_TRADE_SUMMARY_URL); request.setRequestHeader('Content-type', 'application/json'); const partnerSteamID = offer.partner.toString(); const tradeSummary = offer.summarize(this.bot.schema); let tradesTotal = 0; const offerData = this.bot.manager.pollData.offerData; for (const offerID in offerData) { if (!Object.prototype.hasOwnProperty.call(offerData, offerID)) { continue; } if (offerData[offerID].handledByUs === true && offerData[offerID].isAccepted === true) { // Sucessful trades handled by the bot tradesTotal++; } } const tradesMade = process.env.TRADES_MADE_STARTER_VALUE ? +process.env.TRADES_MADE_STARTER_VALUE + tradesTotal : 0 + tradesTotal; let personaName; let avatarFull; getPartnerDetails(offer, function(err, details) { if (err) { log.debug('Error getting partner details, returning ? avatar and unknown name'); personaName = 'unknown'; avatarFull = 'https://p7.hiclipart.com/preview/313/980/1020/question-mark-icon-question-mark-png.jpg'; } else { log.debug('Successfully obtained partner details'); personaName = details.personaName; avatarFull = details.avatarFull; } const stringified = JSON.stringify(discordTradeSummary) .replace(/%partnerId%/g, partnerSteamID) .replace(/%partnerName%/g, partnerName) .replace(/%partnerAvatar%/g, partnerAvatar) .replace(/%offerId%/g, offer.id) .replace(/%tradeNum%/g, tradesMade.toString()) .replace(/%tradeSummary%/g, tradeSummary.replace('Offered:', '\\n Offered:')) .replace(/%currentTime%/g, moment().format('MMMM Do YYYY, HH:mm:ss') + ' UTC'); const jsonObject = JSON.parse(stringified); request.send(JSON.stringify(jsonObject)); }); log.debug('Accepted trade summmary sent to webhook'); } Lemme know if there are any errors, I haven't tested this. -
How to properly use getUserDetails function
vrtgn replied to IdiNium's topic in node-steam-tradeoffer-manager
Your usage of getUserDetails is correct. Here is the documentation for it. There are multiple problems: You are making the variable partnerAvatar and partnerName equal to a function (also known as a void). The error you are receiving is that the logger cannot log functions. You are trying to assign the value of those variables by returning a value from the callback function which is incorrect. You are attempting to log the values outside the callback. You are calling offer.getUserDetails twice which is bad practice. Firstly, you can't log functions, there is no need to, and that is not even what you are trying to do. Secondly, you can assign variables to functions and call them (here are some different ways to make functions), but you can't assign a value to that variable (as it is already a function) from that function. Thirdly, you need to have an understanding of how callbacks work. Basically, when you call offer.getUserDetails it sends steam a request which takes time. When it gets the response, the offer class callbacks to that function. If you log instantly after the callback it will log it as null/undefined. Instead you need to log inside the callback when the details have been received. Finally, if you have already called offer.getUserDetails, there is no reason to call it again. Just get the variables from the first call. This is my solution which would be there instead of lines 1020 - 1056. offer.getUserDetails(function(err, me, them) { if (err) { // handle error, log.debug(err), etc. } else { const partnerAvatar = them.avatarFull; const partnerName = them.personaName; log.debug(partnerAvatar); log.debug(partnerName); const stringified = JSON.stringify(discordReviewOfferSummary) .replace(/%partnerId%/g, partnerSteamID) .replace(/%partnerName%/g, '//Coming Soon//') .replace(/%partnerAvatar%/g, partnerAvatar) .replace(/%offerId%/g, offer.id) .replace(/%reason%/g, reason) .replace(/%tradeSummary%/g, offer.summarize(this.bot.schema).replace('Offered:', '\\n Offered:')) .replace(/%ownerDiscordId%/g, process.env.OWNER_DISCORD_ID) .replace(/%currentTime%/g, moment().format('MMMM Do YYYY, HH:mm:ss') + ' UTC'); const jsonObject = JSON.parse(stringified); request.send(JSON.stringify(jsonObject)); log.debug('Review offer summary sent to webhook'); } }) Hope this helps, and if I'm wrong feel free to correct me -
Thanks for your reply. I guess you could use some combination of marketable/tradable after to work it out, or something like that.
-
If I have buy orders in the steam community market, how can I get their details (asset ids, and how many we bought) when they are bought? Thanks in advance
-
Here's an example: function updateDescription(description) { community.editProfile({ summary: description }, (err) => { if (err) { console.log("Oops. Something went wrong when changing the description"); } else { console.log("Description changed to " + description); } }); } updateDescription("Hi"); // changes description to Hi
-
This isn't the forum for this but Friends/old friends can be seen through here: https://steamid.uk/ (provided there profile is/was public) Inventory can be seen through: https://backpack.tf and https://csgo.backpack.tf.
-
Check this out.
-
You're not meant to use the method I gave in conjunction with the confirmation checker. The confirmation checker (in simple terms) works by telling Steam every X seconds: "Hey, I wanna confirm everything waiting to be confirmed". It will tell steam even if there is nothing waiting to be confirmed thus the load it puts on servers - imagine loads of bots doing this. Whereas the method I put tells steams servers: "Hey, I wanna confirm this specific trade". Therefore, it only tells steam when there is something to be confirmed.
-
Limited accounts are not allowed to prevent overload e.g. say someone makes a bunch of bots and then spams reqeusts to steam - all for free as well. API keys - verify the user requesting is not limited and see/track who is making the calls and how many.
-
Do npm install -g steam-user which installs it globally. Otherwise, you just didn't install it in the first place, like doc said.
-
Using a confirmation checker puts unnecessary load on steam's servers. After receiving or sending a trade offer, obtain the offer's ID and then call this method: community.acceptConfirmationForObject('YOUR_IDENTITY_SECRET', 'OFFER ID', (err) => { if (err) { console.log(err); } // code here })
-
community.on('sessionExpired', function(err) { client.webLogOn(); //relogin to Steam or refresh tradeoffer-manager cookies })
-
Bot crashes immediately after 1 trade
vrtgn replied to SeMaKKa's topic in node-steam-tradeoffer-manager
You aren't friends with the other user and you didn't provide a trade token The provided trade token was wrong You are trying to send or receive an item for a game in which you or the other user can't trade (e.g. due to a VAC ban) You are trying to send an item and the other user's inventory is full for that game -
Your webSession must have expired. community.on('sessionExpired', function(err) { client.webLogOn(); //relogin to Steam or refresh tradeoffer-manager cookies })
- 1 reply
-
- suggestion
- nodejs
-
(and 2 more)
Tagged with:
-
Switch Case Default exclusion Trade Offer Messages
vrtgn replied to ahmet's topic in node-steamcommunity
if (message.includes("[tradeoffer")) { return } Put this before your switch case. -
Look into socket.io.